优化这个代码的性能from Bio import SeqIOfrom Bio.SeqFeature import SeqFeature, FeatureLocation# 读取基因组注释文件annotation_file = "genome_annotation.gff3"annotations = SeqIO.parse(annotation_file, "gff3")# 创建字典用于存储每个基因的最长转录本信息gene_to_longest_transcript = {}# 遍历每个注释项for annotation in annotations: if annotation.type == "mRNA": # 获取基因名和转录本ID gene_name = annotation.qualifiers.get("gene") transcript_id = annotation.qualifiers.get("transcript_id") if gene_name and transcript_id: gene_name = gene_name[0] transcript_id = transcript_id[0] # 检查之前是否已经处理该基因,并选择最长的转录本 if gene_name in gene_to_longest_transcript: longest_transcript = gene_to_longest_transcript[gene_name] if len(annotation) > len(longest_transcript): gene_to_longest_transcript[gene_name] = annotation else: gene_to_longest_transcript[gene_name] = annotation# 打印每个基因的最长转录本信息for gene_name, longest_transcript in gene_to_longest_transcript.items(): print(gene_name, longest_transcript.id, len(longest_transcript))
时间: 2023-06-04 13:04:42 浏览: 51
这个代码可以通过以下几个步骤来优化性能:
1. 尽可能使用内置的函数和数据结构,比如使用 list comprehension 而不是 for 循环来遍历序列。
2. 减少冗余的计算。可以使用缓存来存储计算结果,在需要的时候直接获取缓存中的值,而不是重新计算。
3. 使用适当的数据结构来处理大规模数据,比如使用 set 而不是 list 来存储大量的元素。
4. 将代码分解成更小的模块,以便于调试和优化。
5. 减少 I/O 操作的次数,将数据读取到内存中后就尽可能多地处理,而不是每次都从文件中读取。
6. 使用并行处理来提高性能,比如使用多进程或多线程来同时处理多个任务。
相关问题
优化这个代码from Bio import SeqIOfrom Bio.SeqFeature import SeqFeature, FeatureLocation# 读取基因组注释文件annotation_file = "genome_annotation.gff3"annotations = SeqIO.parse(annotation_file, "gff3")# 创建字典用于存储每个基因的最长转录本信息gene_to_longest_transcript = {}# 遍历每个注释项for annotation in annotations: if annotation.type == "mRNA": # 获取基因名和转录本ID gene_name = annotation.qualifiers.get("gene") transcript_id = annotation.qualifiers.get("transcript_id") if gene_name and transcript_id: gene_name = gene_name[0] transcript_id = transcript_id[0] # 检查之前是否已经处理该基因,并选择最长的转录本 if gene_name in gene_to_longest_transcript: longest_transcript = gene_to_longest_transcript[gene_name] if len(annotation) > len(longest_transcript): gene_to_longest_transcript[gene_name] = annotation else: gene_to_longest_transcript[gene_name] = annotation# 打印每个基因的最长转录本信息for gene_name, longest_transcript in gene_to_longest_transcript.items(): print(gene_name, longest_transcript.id, len(longest_transcript))
优化这个代码的方法有很多,可以从以下几个方面入手:
1. 代码可读性与可维护性:可以添加注释,规范命名等,让代码更易读懂,并方便其他人对代码的修改与维护。
2. 代码性能:可以使用一些优化技巧,如使用map(), filter()等高阶函数来优化代码性能。
3. 代码结构:可以将代码按照功能进行分块,增加代码的可重用性,例如可以将不同功能的代码封装成函数。
4. 引入第三方库:可以利用一些现成的优化工具,如numpy,pandas等库,来简化代码、提高代码性能。
总之,优化代码需要针对具体问题具体分析,从不同方向出发,寻找最优解。
from Bio import SeqIOfrom Bio.SeqFeature import SeqFeature, FeatureLocation# 读取基因组注释文件annotation_file = "genome_annotation.gff3"annotations = SeqIO.parse(annotation_file, "gff3")# 创建字典用于存储每个基因的最长转录本信息gene_to_longest_transcript = {}# 遍历每个注释项for annotation in annotations: if annotation.type == "mRNA": # 获取基因名和转录本ID gene_name = annotation.qualifiers.get("gene") transcript_id = annotation.qualifiers.get("transcript_id") if gene_name and transcript_id: gene_name = gene_name[0] transcript_id = transcript_id[0] # 检查之前是否已经处理该基因,并选择最长的转录本 if gene_name in gene_to_longest_transcript: longest_transcript = gene_to_longest_transcript[gene_name] if len(annotation) > len(longest_transcript): gene_to_longest_transcript[gene_name] = annotation else: gene_to_longest_transcript[gene_name] = annotation# 打印每个基因的最长转录本信息for gene_name, longest_transcript in gene_to_longest_transcript.items(): print(gene_name, longest_transcript.id, len(longest_transcript))
这个是Python中Bio模块的导入语句。其中SeqIO和SeqFeature分别是Bio模块中的两个子模块,用于序列文件的读取和序列特征的处理。SeqFeature中的FeatureLocation则是用于指定序列特征位置的工具。
相关推荐
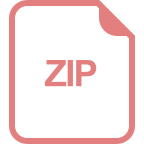
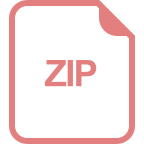













