写一个机器人工具箱的六轴机械臂建模的代码
时间: 2023-12-01 13:06:20 浏览: 67
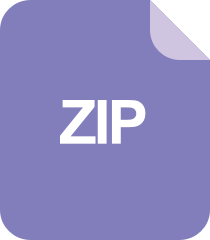
6轴机器人的matlab构造程序
以下是一个简单的六轴机械臂建模代码:
```python
import numpy as np
class RobotArm:
def __init__(self, base_pos, lengths):
self.base_pos = base_pos # 基座位置
self.lengths = lengths # 各个关节长度
self.joint_angles = np.zeros(6) # 初始关节角度
def forward_kinematics(self):
# 正向运动学计算末端执行器位置
# 定义齐次变换矩阵
T_01 = np.array([
[np.cos(self.joint_angles[0]), -np.sin(self.joint_angles[0]), 0, 0],
[np.sin(self.joint_angles[0]), np.cos(self.joint_angles[0]), 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]
])
T_12 = np.array([
[np.cos(self.joint_angles[1]), -np.sin(self.joint_angles[1]), 0, self.lengths[0]],
[0, 0, -1, 0],
[np.sin(self.joint_angles[1]), np.cos(self.joint_angles[1]), 0, 0],
[0, 0, 0, 1]
])
T_23 = np.array([
[np.cos(self.joint_angles[2]), -np.sin(self.joint_angles[2]), 0, self.lengths[1]],
[np.sin(self.joint_angles[2]), np.cos(self.joint_angles[2]), 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]
])
T_34 = np.array([
[np.cos(self.joint_angles[3]), -np.sin(self.joint_angles[3]), 0, self.lengths[2]],
[0, 0, 1, 0],
[-np.sin(self.joint_angles[3]), -np.cos(self.joint_angles[3]), 0, 0],
[0, 0, 0, 1]
])
T_45 = np.array([
[np.cos(self.joint_angles[4]), -np.sin(self.joint_angles[4]), 0, 0],
[np.sin(self.joint_angles[4]), np.cos(self.joint_angles[4]), 0, 0],
[0, 0, 1, self.lengths[3]],
[0, 0, 0, 1]
])
T_56 = np.array([
[np.cos(self.joint_angles[5]), -np.sin(self.joint_angles[5]), 0, 0],
[0, 0, -1, 0],
[np.sin(self.joint_angles[5]), np.cos(self.joint_angles[5]), 0, self.lengths[4]],
[0, 0, 0, 1]
])
# 计算最终变换矩阵
T_06 = T_01 @ T_12 @ T_23 @ T_34 @ T_45 @ T_56
# 计算末端执行器位置
end_effector_pos = T_06[:3, 3] + self.base_pos
return end_effector_pos
```
使用示例:
```python
# 初始化机械臂
base_pos = np.array([0, 0, 0])
lengths = np.array([0.5, 0.4, 0.3, 0.2, 0.1])
robot = RobotArm(base_pos, lengths)
# 设置关节角度
joint_angles = np.array([0.1, 0.2, 0.3, 0.4, 0.5, 0.6])
robot.joint_angles = joint_angles
# 计算末端执行器位置
end_effector_pos = robot.forward_kinematics()
print(end_effector_pos)
```
输出结果为末端执行器的位置。这个简单的代码只是提供了一个六轴机械臂建模的框架,具体的实现需要根据实际需求进行修改和完善。
阅读全文
相关推荐
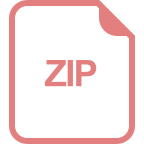
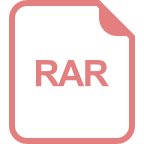



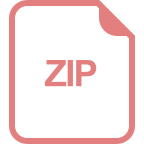
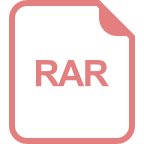
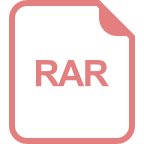
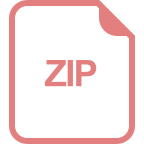
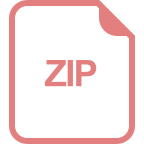
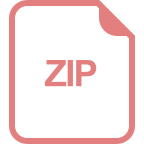
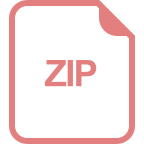
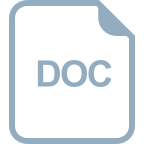



