查询一段时间间隔内的数据的mysql语句
时间: 2023-09-22 09:07:44 浏览: 62
好的,以下是一个查询 MySQL 数据库中表格数据在时间间隔内的 SQL 语句示例:
```
SELECT * FROM table_name WHERE date_column BETWEEN start_date AND end_date;
```
其中,`table_name` 是您想要查询的表格名称,`date_column` 是表格中表示时间的列名,`start_date` 和 `end_date` 是您想要查询的时间间隔的起始日期和结束日期。
请注意,这个语句可以用于查询日期或日期时间类型的列。如果您的列类型是时间戳类型,则需要使用 `FROM_UNIXTIME()` 函数将时间戳转换为日期时间格式。
希望以上的回答能够帮到您。
相关问题
查询固定时间间隔内的数据
要查询固定时间间隔内的数据,你需要使用数据库或其他数据存储系统提供的查询功能。具体的查询语句会因不同的系统而异,但通常都会涉及到WHERE子句和日期时间函数。
例如,如果你使用MySQL数据库,可以使用如下的查询语句来查询过去一周内的数据:
```
SELECT * FROM table_name
WHERE date_column >= DATE_SUB(NOW(), INTERVAL 1 WEEK);
```
这个查询语句中,table_name是你要查询的表的名称,date_column是存储日期时间的列的名称。NOW()函数返回当前日期时间,DATE_SUB()函数可以将一段时间从当前日期时间中减去,从而得到过去一周的日期时间。WHERE子句用于筛选符合条件的数据行。
你需要根据你所使用的具体数据存储系统和数据结构,来编写相应的查询语句。
mysql 一段时间内每隔十分钟生成时间的一列的sql
假设你需要在MySQL中生成一列时间数据,这列数据包含了某个时间段内每隔十分钟的时间点,可以使用以下SQL语句:
```
SELECT TIMESTAMPADD(MINUTE, (t*10), '2021-08-01 00:00:00') AS time
FROM (
SELECT 0 AS t UNION SELECT 1 UNION SELECT 2 UNION SELECT 3 UNION SELECT 4 UNION
SELECT 5 UNION SELECT 6 UNION SELECT 7 UNION SELECT 8 UNION SELECT 9 UNION
SELECT 10 UNION SELECT 11 UNION SELECT 12 UNION SELECT 13 UNION SELECT 14 UNION
SELECT 15 UNION SELECT 16 UNION SELECT 17 UNION SELECT 18 UNION SELECT 19 UNION
SELECT 20 UNION SELECT 21 UNION SELECT 22 UNION SELECT 23 UNION SELECT 24 UNION
SELECT 25 UNION SELECT 26 UNION SELECT 27 UNION SELECT 28 UNION SELECT 29
) AS minutes
WHERE
TIMESTAMPADD(MINUTE, (t*10), '2021-08-01 00:00:00') BETWEEN '2021-08-01 00:00:00' AND '2021-08-02 00:00:00'
```
这条SQL语句会生成一个包含指定时间段内每隔十分钟的时间点的数据集合。其中,`TIMESTAMPADD`函数会返回一个日期时间值,在指定的时间值上加上一定的时间间隔,这里我们每次加10分钟。`SELECT`语句中的子查询会生成0到29的数字序列,每个数字乘以10,最终生成0到290之间的数字集合,这些数字代表了时间点与起始时间点的时间差。`WHERE`子句中的条件会过滤掉指定时间范围之外的时间点。
你可以根据实际需求修改SQL语句中的起始时间点、时间间隔和查询时间范围。
相关推荐
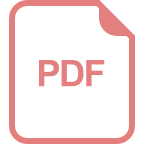
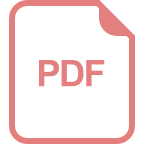
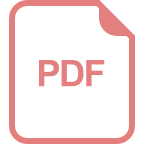












