spring Mvc 6.0.9 中文件上传和下载怎么做?
时间: 2024-03-19 08:45:24 浏览: 67
实现文件上传和下载在Spring MVC中非常简单,下面是具体的实现步骤:
文件上传:
1. 在Spring配置文件中添加以下bean配置:
```xml
<bean id="multipartResolver"
class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8" />
<property name="maxUploadSize" value="10485760" />
</bean>
```
其中,`defaultEncoding`属性用于设置上传文件的编码格式,`maxUploadSize`属性用于设置最大上传文件的大小。
2. 在Controller中添加文件上传方法:
```java
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public String upload(@RequestParam("file") MultipartFile file) {
if (!file.isEmpty()) {
String fileName = file.getOriginalFilename();
String filePath = "/path/to/save/" + fileName;
try {
file.transferTo(new File(filePath));
return "上传成功!";
} catch (IOException e) {
e.printStackTrace();
}
}
return "上传失败,请选择文件!";
}
```
其中,`@RequestParam`用于获取上传的文件,`file`是表单中文件上传控件的name属性值。
文件下载:
1. 在Controller中添加文件下载方法:
```java
@RequestMapping(value = "/download", method = RequestMethod.GET)
public ResponseEntity<byte[]> download() throws IOException {
String filePath = "/path/to/file";
File file = new File(filePath);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", file.getName());
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file), headers, HttpStatus.CREATED);
}
```
其中,`filePath`为要下载的文件路径,`FileUtils.readFileToByteArray(file)`用于将文件转换成字节数组。
2. 在JSP页面中添加下载链接:
```html
<a href="/download">下载文件</a>
```
至此,完成了Spring MVC中文件上传和下载的实现。
阅读全文
相关推荐
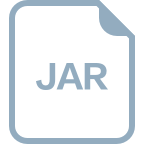

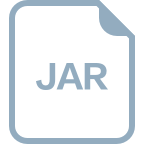















