用pygame库做一个双人贪吃蛇的代码
时间: 2024-05-11 17:16:40 浏览: 224
好的,以下是用pygame库做一个双人贪吃蛇的代码:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 游戏区域的大小
screen_width = 600
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("双人贪吃蛇")
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
green = (0, 255, 0)
# 定义贪吃蛇的类
class Snake():
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.direction = "RIGHT"
self.length = 1
self.body = []
self.body.append([self.x, self.y])
# 移动
def move(self):
if self.direction == "UP":
self.y -= 10
elif self.direction == "DOWN":
self.y += 10
elif self.direction == "LEFT":
self.x -= 10
elif self.direction == "RIGHT":
self.x += 10
# 检查是否吃到食物
def eat_food(self, food):
if self.x == food.x and self.y == food.y:
self.length += 1
self.body.append([self.x, self.y])
food.generate()
# 死亡检查
def die(self):
if self.x < 0 or self.x > screen_width or self.y < 0 or self.y > screen_height:
return True
for block in self.body[1:]:
if self.x == block[0] and self.y == block[1]:
return True
return False
# 更新身体
def update_body(self):
self.body.append([self.x, self.y])
if len(self.body) > self.length:
del self.body[0]
# 绘制贪吃蛇
def draw(self):
for block in self.body:
pygame.draw.rect(screen, self.color, [block[0], block[1], 10, 10])
# 定义食物的类
class Food():
def __init__(self, color):
self.color = color
self.x = round(random.randrange(0, screen_width - 10) / 10.0) * 10.0
self.y = round(random.randrange(0, screen_height - 10) / 10.0) * 10.0
# 生成食物
def generate(self):
self.x = round(random.randrange(0, screen_width - 10) / 10.0) * 10.0
self.y = round(random.randrange(0, screen_height - 10) / 10.0) * 10.0
# 绘制食物
def draw(self):
pygame.draw.rect(screen, self.color, [self.x, self.y, 10, 10])
# 初始化贪吃蛇和食物
snake1 = Snake(100, 100, red)
snake2 = Snake(500, 500, green)
food = Food(black)
# 游戏循环
game_over = False
clock = pygame.time.Clock()
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake1.direction = "UP"
elif event.key == pygame.K_DOWN:
snake1.direction = "DOWN"
elif event.key == pygame.K_LEFT:
snake1.direction = "LEFT"
elif event.key == pygame.K_RIGHT:
snake1.direction = "RIGHT"
elif event.key == pygame.K_w:
snake2.direction = "UP"
elif event.key == pygame.K_s:
snake2.direction = "DOWN"
elif event.key == pygame.K_a:
snake2.direction = "LEFT"
elif event.key == pygame.K_d:
snake2.direction = "RIGHT"
# 清除屏幕
screen.fill(white)
# 移动贪吃蛇
snake1.move()
snake2.move()
# 检查是否吃到食物
snake1.eat_food(food)
snake2.eat_food(food)
# 更新贪吃蛇的身体
snake1.update_body()
snake2.update_body()
# 绘制贪吃蛇和食物
snake1.draw()
snake2.draw()
food.draw()
# 检查是否死亡
if snake1.die() or snake2.die():
game_over = True
# 更新屏幕
pygame.display.update()
# 控制游戏帧率
clock.tick(20)
# 退出游戏
pygame.quit()
quit()
```
希望能够帮到你!
阅读全文
相关推荐















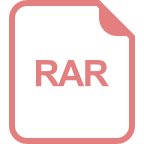