java 合并多个docx文件
时间: 2023-09-08 18:17:09 浏览: 42
要合并多个docx文件,可以使用Java的Apache POI库。以下是一个简单的示例代码,可以将多个docx文件合并成一个新的docx文件:
```
import java.io.*;
import org.apache.poi.xwpf.usermodel.*;
public class MergeDocxFiles {
public static void main(String[] args) throws Exception {
// 读取所有要合并的文档
XWPFDocument[] docs = new XWPFDocument[3];
for (int i = 0; i < docs.length; i++) {
FileInputStream in = new FileInputStream("doc" + i + ".docx");
docs[i] = new XWPFDocument(in);
if (i > 0) {
// 将后面的文档内容添加到第一个文档中
appendDoc(docs[0], docs[i]);
}
in.close();
}
// 将合并后的文档保存到新文件中
FileOutputStream out = new FileOutputStream("merged.docx");
docs[0].write(out);
out.close();
}
// 将一个文档的内容添加到另一个文档的末尾
private static void appendDoc(XWPFDocument doc1, XWPFDocument doc2) throws Exception {
for (XWPFParagraph para : doc2.getParagraphs()) {
XWPFParagraph newPara = doc1.createParagraph();
newPara.createRun().setText(para.getText());
}
for (XWPFTable table : doc2.getTables()) {
XWPFTable newTable = doc1.createTable();
newTable.getCTTbl().setTblPr(table.getCTTbl().getTblPr());
for (int i = 0; i < table.getRows().size(); i++) {
XWPFTableRow newRow = newTable.createRow();
newRow.getCtRow().setTrPr(table.getRow(i).getCtRow().getTrPr());
for (int j = 0; j < table.getRow(i).getTableCells().size(); j++) {
XWPFTableCell newCell = newRow.createCell();
newCell.getCTTc().setTcPr(table.getRow(i).getCell(j).getCTTc().getTcPr());
for (XWPFParagraph para : table.getRow(i).getCell(j).getParagraphs()) {
XWPFParagraph newPara = newCell.addParagraph();
newPara.createRun().setText(para.getText());
}
}
}
}
}
}
```
这个示例代码可以读取名为`doc0.docx`、`doc1.docx`和`doc2.docx`的三个文档,将它们合并成一个新的docx文件`merged.docx`。如果要合并更多的文档,只需要改变`docs`数组的大小和文件名即可。
相关推荐
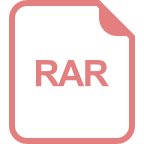













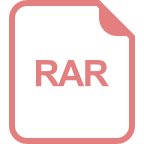
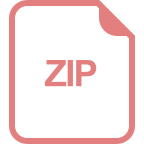