Python编写一个能读写json文件的程序
时间: 2023-05-29 15:02:24 浏览: 128
以下是一个能读写json文件的Python程序示例:
```python
import json
# 读取json文件
with open('data.json', 'r', encoding='utf-8') as f:
data = json.load(f)
# 输出读取的数据
print(data)
# 修改数据
data['name'] = 'Tom'
data['age'] = 25
# 写入json文件
with open('data.json', 'w', encoding='utf-8') as f:
json.dump(data, f)
# 再次读取json文件
with open('data.json', 'r', encoding='utf-8') as f:
data = json.load(f)
# 输出修改后的数据
print(data)
```
在此示例中,我们首先打开一个名为“data.json”的json文件进行读取,然后将读取到的数据存储在变量“data”中。接着,我们修改了其中的一些数据,并将其写回到同一个文件中。最后,我们再次读取文件,并输出修改后的数据。注意,我们使用了`json.load()`函数读取json文件,`json.dump()`函数将数据写回json文件。
相关问题
python json模块
Python 的 `json` 模块提供了对 JSON(JavaScript Object Notation)数据的编码和解码功能。JSON 是一种轻量级的数据交换格式,易于阅读和编写,也易于机器解析和生成。在 Python 中,我们可以通过 `json` 模块来进行 JSON 数据的读写操作。
下面是一个简单的例子,演示了如何将 Python 对象编码成 JSON 数据,以及如何将 JSON 数据解码成 Python 对象:
```python
import json
# 将 Python 对象编码成 JSON 数据
data = {
'name': 'John',
'age': 30,
'city': 'New York'
}
json_data = json.dumps(data)
print(json_data)
# 将 JSON 数据解码成 Python 对象
decoded_data = json.loads(json_data)
print(decoded_data)
```
输出结果:
```
{"name": "John", "age": 30, "city": "New York"}
{'name': 'John', 'age': 30, 'city': 'New York'}
```
在上面的代码中,`json.dumps()` 函数将 Python 对象 `data` 编码成 JSON 数据,并将结果存储在变量 `json_data` 中。`json.loads()` 函数将 JSON 数据解码成 Python 对象,并将结果存储在变量 `decoded_data` 中。
除了上面的 `dumps()` 和 `loads()` 函数,`json` 模块还提供了其他一些函数和类,用于更高级的 JSON 数据处理。具体可以参考 Python 官方文档。
编写flask代码对postgres数据库进行JSON格式数据的读写操作
好的,我可以为您提供一些关于使用Flask对PostgreSQL数据库进行JSON格式数据读写操作的代码示例。
在使用Flask和PostgreSQL之前,您需要先安装所需的Python包。请使用以下命令安装:
```
pip install Flask psycopg2-binary
```
接下来,您可以按照以下步骤来编写Flask代码以读写PostgreSQL中的JSON数据:
1. 导入所需的包:
```python
from flask import Flask, jsonify, request
import psycopg2
```
2. 建立PostgreSQL数据库连接:
```python
conn = psycopg2.connect(database="your_database_name", user="your_username", password="your_password", host="your_host", port="your_port")
```
3. 创建Flask应用程序:
```python
app = Flask(__name__)
```
4. 编写一个函数,用于从PostgreSQL数据库中读取JSON格式数据:
```python
@app.route('/get_json_data')
def get_json_data():
cur = conn.cursor()
cur.execute("SELECT json_data FROM your_table_name")
rows = cur.fetchall()
data = []
for row in rows:
data.append(row[0])
cur.close()
return jsonify(data)
```
5. 编写一个函数,用于将JSON格式数据写入PostgreSQL数据库:
```python
@app.route('/write_json_data', methods=['POST'])
def write_json_data():
json_data = request.json
cur = conn.cursor()
cur.execute("INSERT INTO your_table_name (json_data) VALUES (%s)", (json_data,))
conn.commit()
cur.close()
return jsonify({"message": "Data written successfully"})
```
6. 运行Flask应用程序:
```python
if __name__ == '__main__':
app.run()
```
这样,您就可以使用Flask和PostgreSQL来读写JSON格式数据了。请记得将代码中的数据库连接信息替换为您自己的信息。
相关推荐
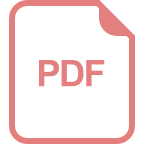
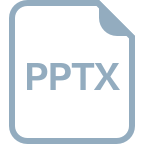
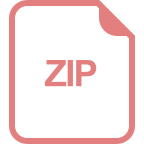












