用java写一个easy excle导入excel功能代码
时间: 2023-07-15 08:12:58 浏览: 65
以下是一个简单的Java代码示例,用于将Excel文件中的数据导入到数据库中:
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelImport {
public static void main(String[] args) {
String jdbcURL = "jdbc:mysql://localhost:3306/mydatabase";
String username = "username";
String password = "password";
String excelFilePath = "data.xlsx";
int batchSize = 20;
Connection connection = null;
try {
long start = System.currentTimeMillis();
FileInputStream inputStream = new FileInputStream(new File(excelFilePath));
Workbook workbook = WorkbookFactory.create(inputStream);
Sheet sheet = workbook.getSheetAt(0);
connection = DriverManager.getConnection(jdbcURL, username, password);
connection.setAutoCommit(false);
String sql = "INSERT INTO students (name, email, phone) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
int count = 0;
for (Row row : sheet) {
if (count == 0) {
count++;
continue;
}
String name = row.getCell(0).getStringCellValue();
String email = row.getCell(1).getStringCellValue();
String phone = row.getCell(2).getStringCellValue();
statement.setString(1, name);
statement.setString(2, email);
statement.setString(3, phone);
statement.addBatch();
if (count % batchSize == 0) {
statement.executeBatch();
}
}
workbook.close();
statement.executeBatch();
connection.commit();
connection.close();
long end = System.currentTimeMillis();
System.out.printf("Import done in %d ms\n", (end - start));
} catch (Exception e) {
e.printStackTrace();
try {
connection.rollback();
} catch (SQLException e1) {
System.out.println(e1.getMessage());
}
}
}
}
```
这个示例代码使用了Apache POI库来读取Excel文件,并使用JDBC连接到MySQL数据库。对于每一行数据,它会将数据插入到“ students”表中。您需要将代码中的“ mydatabase”、“ username”和“ password”替换为您的数据库名称、用户名和密码。同时,您还需要将代码中的“ data.xlsx”替换为您的Excel文件路径。
相关推荐
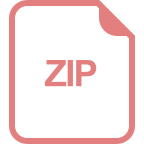















