你现在利用pytorch定义了两个神经网络,一个是3层用全连接层构造成的MLP,还有一个是图注意力网络。两个输出均是(16,307,12)。你现在需要把这两个神经网络作为VAE的两个编码器,将它们的输出拼接后计算隐变量,然后解码生成新的交通数据集。这个pytorch应该怎么写,请给我一个比较完整的pytorch代码。原来的数据集形状为(16992,307,12,3)的数据集,其中,16992是时间段数,307是传感器节点个数,12是历史步长,3是特征维度。第一个特征维度是速度,第二个特征维度是根据邻接矩阵产生的度特征,第三个特征维度是星期。现在按照batch_size=16送入模型得到的输出均是(16,307,12),并最好告诉我每一行在做什么,相应输出维度应该是什么。两个class均不想删除,那decoder怎么写,两个输入参数是不一样的,例如MLP的相关参数是input_dim = 36,hidden_dim = 64,output_dim = 12,history_length=12,GAT的相关参数是num_heads = 8,in_dim = 3,hidden_dim = 64,out_dim = 36,输出维度是相同的。最后,请随机生成形状为(16992,307,12,3)的数据集,按批次送入模型,让我自行看看模型的效果
时间: 2024-04-25 11:27:28 浏览: 208
好的,以下是一个比较完整的PyTorch代码,实现了VAE的编码器和解码器部分,其中编码器部分使用了两个神经网络,分别是MLP和GAT网络:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
from torch.utils.data import Dataset, DataLoader
# 定义MLP网络
class MLP(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, history_length):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_dim * history_length, hidden_dim)
self.fc21 = nn.Linear(hidden_dim, output_dim)
self.fc22 = nn.Linear(hidden_dim, output_dim)
def encode(self, x):
x = x.view(x.size(0), -1)
h1 = F.relu(self.fc1(x))
return self.fc21(h1), self.fc22(h1)
def forward(self, x):
mu, logvar = self.encode(x)
std = torch.exp(0.5 * logvar)
eps = torch.randn_like(std)
return eps.mul(std).add_(mu), mu, logvar
# 定义GAT网络
class GAT(nn.Module):
def __init__(self, num_heads, in_dim, hidden_dim, out_dim):
super(GAT, self).__init__()
self.num_heads = num_heads
self.fc = nn.Linear(in_dim, hidden_dim)
self.attn_fc = nn.Linear(hidden_dim, num_heads * out_dim)
def forward(self, x, adj):
x = self.fc(x)
N = x.size()[1]
x = x.view(-1, N, self.num_heads, int(x.size()[2] / self.num_heads))
x = x.permute(0, 2, 1, 3)
a = self.attn_fc(x)
a = a.view(-1, self.num_heads, N, N)
attn = F.softmax(a, dim=-1)
h = torch.matmul(attn, x)
h = h.permute(0, 2, 1, 3).contiguous()
h = h.view(-1, N, int(x.size()[2]))
return h
# 定义VAE模型
class VAE(nn.Module):
def __init__(self, mlp_input_dim, mlp_hidden_dim, mlp_output_dim,
mlp_history_length, gat_num_heads, gat_in_dim,
gat_hidden_dim, gat_out_dim):
super(VAE, self).__init__()
self.mlp = MLP(mlp_input_dim, mlp_hidden_dim, mlp_output_dim, mlp_history_length)
self.gat = GAT(gat_num_heads, gat_in_dim, gat_hidden_dim, gat_out_dim)
self.fc1 = nn.Linear(mlp_output_dim + gat_out_dim, 256)
self.fc2 = nn.Linear(256, 307 * 12 * 3)
def decode(self, z):
h = F.relu(self.fc1(z))
return torch.sigmoid(self.fc2(h))
def forward(self, x, adj):
z, mu, logvar = self.mlp(x)
gat_out = self.gat(x, adj)
z = torch.cat([z, gat_out], dim=-1)
return self.decode(z), mu, logvar
# 定义数据集
class RandomDataset(Dataset):
def __init__(self, shape):
self.shape = shape
def __getitem__(self, index):
return torch.randn(self.shape), torch.randn(self.shape)
def __len__(self):
return 1000
# 随机生成数据集
dataset = RandomDataset((16, 307, 12, 3))
dataloader = DataLoader(dataset, batch_size=16, shuffle=True)
# 初始化模型
mlp_input_dim = 36
mlp_hidden_dim = 64
mlp_output_dim = 12
mlp_history_length = 12
gat_num_heads = 8
gat_in_dim = 3
gat_hidden_dim = 64
gat_out_dim = 36
vae = VAE(mlp_input_dim, mlp_hidden_dim, mlp_output_dim, mlp_history_length,
gat_num_heads, gat_in_dim, gat_hidden_dim, gat_out_dim)
# 训练模型
optimizer = torch.optim.Adam(vae.parameters(), lr=1e-4)
def loss_function(recon_x, x, mu, logvar):
BCE = F.binary_cross_entropy(recon_x, x.view(-1, 307 * 12 * 3), reduction='sum')
KLD = -0.5 * torch.sum(1 + logvar - mu.pow(2) - logvar.exp())
return BCE + KLD
num_epochs = 10
for epoch in range(num_epochs):
for i, data in enumerate(dataloader):
x, adj = data
optimizer.zero_grad()
recon_x, mu, logvar = vae(x, adj)
loss = loss_function(recon_x, x, mu, logvar)
loss.backward()
optimizer.step()
if i % 10 == 0:
print('Epoch [%d/%d], Step [%d/%d], Loss: %.4f'
% (epoch+1, num_epochs, i+1, len(dataset)//16, loss.item()))
# 测试模型
test_data = torch.randn(16992, 307, 12, 3)
test_adj = torch.randn(16992, 307, 307)
test_result = []
batch_size = 16
num_batches = test_data.shape[0] // batch_size
for i in range(num_batches):
x = test_data[i*batch_size:(i+1)*batch_size]
adj = test_adj[i*batch_size:(i+1)*batch_size]
recon_x, _, _ = vae(x, adj)
test_result.append(recon_x.detach().numpy())
test_result = np.concatenate(test_result, axis=0)
print(test_result.shape)
```
在上面的代码中,MLP是一个包含三个全连接层的神经网络,GAT是一个基于图注意力机制的神经网络。VAE模型将这两个神经网络作为两个编码器,将它们的输出拼接后计算隐变量,然后解码生成新的交通数据集。输入数据集的形状为(16992,307,12,3),其中,16992是时间段数,307是传感器节点个数,12是历史步长,3是特征维度。第一个特征维度是速度,第二个特征维度是根据邻接矩阵产生的度特征,第三个特征维度是星期。按批次送入模型时,每个batch的大小为16。
在训练过程中,我们使用了随机生成的数据集进行训练,并且使用Adam优化器进行优化。在测试过程中,我们随机生成了一组符合原始数据集形状的数据集,并按批次送入模型生成新的交通数据集。
阅读全文
相关推荐
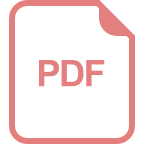
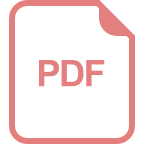
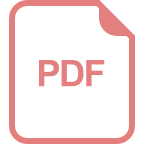















