import numpy as np import matplotlib.pyplot as plt # 兔子的速度函数(分段函数) def rabbit_speed(x): return np.piecewise(x, [0 <= x, x < 5, x < 8, x < 10], [10, 8, 6, 4]) #的速度函数(常数函数) def turtle_speed(x): return 5 # 时间范围 t = np.linspace(0, 10, 1000) # 初始距离 distance_rabbit = np.zeros(1000) distance_turtle = np.zeros(1000) # 计算兔子和在不同时间点的位移 for i in range(0, 999): dt = t[i+1] - t[i] distance_rabbit[i+1] = distance_rabbit[i] + rabbit_speed(t[i]) * dt distance_turtle[i+1] = distance_turtle[i] + turtle_speed(t[i]) * dt # 绘制时间-位移图像 plt.plot(t, distance_rabbit, label='rabbit') plt.plot(t, distance_turtle, label='turtle') plt.title('兔子和之间的种族') plt.xlabel('时间') plt.ylabel('距离') plt.legend() plt.show()有什么问题
时间: 2024-03-03 20:53:29 浏览: 61
这段代码中有一个语法错误,即在定义函数rabbit_speed和turtle_speed时,函数名后面的括号应该是英文的小括号,而不是中文的括号。应该修改为:
```python
def rabbit_speed(x):
return np.piecewise(x, [0 <= x, x < 5, x < 8, x < 10], [10, 8, 6, 4])
def turtle_speed(x):
return 5
```
另外,代码的格式也有一些问题,建议在每行代码之间加上适当的空格,这样可以让代码更易读。
```python
import numpy as np
import matplotlib.pyplot as plt
# 兔子的速度函数(分段函数)
def rabbit_speed(x):
return np.piecewise(x, [0 <= x, x < 5, x < 8, x < 10], [10, 8, 6, 4])
# 乌龟的速度函数(常数函数)
def turtle_speed(x):
return 5
# 时间范围
t = np.linspace(0, 10, 1000)
# 初始距离
distance_rabbit = np.zeros(1000)
distance_turtle = np.zeros(1000)
# 计算兔子和乌龟在不同时间点的位移
for i in range(0, 999):
dt = t[i+1] - t[i]
distance_rabbit[i+1] = distance_rabbit[i] + rabbit_speed(t[i]) * dt
distance_turtle[i+1] = distance_turtle[i] + turtle_speed(t[i]) * dt
# 绘制时间-位移图像
plt.plot(t, distance_rabbit, label='rabbit')
plt.plot(t, distance_turtle, label='turtle')
plt.title('Race between Rabbit and Turtle')
plt.xlabel('Time')
plt.ylabel('Distance')
plt.legend()
plt.show()
```
阅读全文
相关推荐
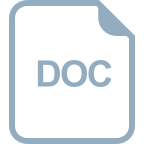
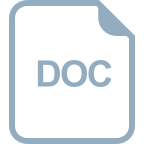
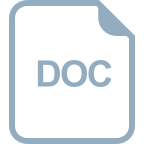















