react-modal 修改模态框遮罩样式
时间: 2023-08-06 10:07:56 浏览: 239
要修改 `react-modal` 模态框的遮罩层样式,可以通过样式对象中的 `overlay` 属性来实现。`overlay` 属性用于设置模态框的遮罩层样式。
例如,你可以在 `customStyles` 对象中添加以下样式来修改遮罩层的背景颜色和透明度:
```jsx
const customStyles = {
overlay: {
backgroundColor: "rgba(0, 0, 0, 0.5)",
zIndex: 100
},
content: {
// ...
}
};
```
在上面的例子中,我们将遮罩层的背景颜色设置为半透明的黑色,透明度为 50%。`zIndex` 属性用于设置遮罩层的层级,确保它位于其他内容之上。
然后,将 `customStyles` 对象传递给 `ReactModal` 组件的 `style` 属性即可:
```jsx
<ReactModal
isOpen={modalIsOpen}
onRequestClose={closeModal}
style={customStyles}
>
<h2>Hello Modal</h2>
<button onClick={closeModal}>Close</button>
</ReactModal>
```
这样就可以修改 `react-modal` 的遮罩层样式了。
相关问题
import ReactModal from 'react-modal'; import './styles.css'; function MyModal() { return ( <ReactModal isOpen={true} overlayClassName="transparent-overlay" > {/* 模态框内容 */} </ReactModal> ); } .transparent-overlay { position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.5); z-index: 999; } 缩小这个模态框的大小,且周围任然不可被点击
为了缩小模态框的大小并且保持周围不可被点击,你可以在ReactModal组件中设置contentClassName属性,并在CSS中设置该类的样式,如下所示:
```
import ReactModal from 'react-modal';
import './styles.css';
function MyModal() {
return (
<ReactModal
isOpen={true}
overlayClassName="transparent-overlay"
contentClassName="custom-modal"
>
{/* 模态框内容 */}
</ReactModal>
);
}
.transparent-overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
z-index: 999;
}
.custom-modal {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50%;
height: 50%;
background-color: white;
z-index: 1000;
}
```
在上面的代码中,我们添加了`contentClassName="custom-modal"`属性,并在CSS中设置`.custom-modal`的样式,使得模态框在页面中垂直和水平居中,且宽度和高度都是50%。同时,我们还将模态框的z-index设置为1000,以确保它始终在遮罩层之上。
import React, { useState } from 'react' import Modal from 'react-modal' function CellClickModal(props) { const value = props.ModalState const [modalIsOpen, setModalIsOpen] = useState(value) function handleClick() { setModalIsOpen(!modalIsOpen) } const customStyles = { overlay: { backgroundColor: 'rgba(0, 0, 0, 0.1)', // 设置透明度为 0.5 的黑色遮罩 }, content: { width: '50%', height: '50%', top: '25%', left: '25%', padding: '20px', }, } return ( <div> <Modal isOpen={modalIsOpen} style={customStyles}> <button onClick={handleClick}>111</button> </Modal> </div> ) } export default CellClickModal
这段代码是使用 React 和 Modal 组件实现的一个弹窗组件,可以通过 props 控制弹窗的状态。useState Hook 用于定义弹窗的状态变量 modalIsOpen 和其更新函数 setModalIsOpen,初始状态值为 props.ModalState。handleClick 函数用于在按钮点击时更新 modalIsOpen 状态值,实现弹窗的打开和关闭。customStyles 对象定义了弹窗的样式,包括遮罩层和弹窗层,可以通过修改其中的属性值来自定义弹窗的外观。最后,Modal 组件渲染了一个按钮和一个弹窗,通过 isOpen 属性值来控制弹窗的显示和隐藏。
阅读全文
相关推荐
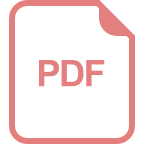
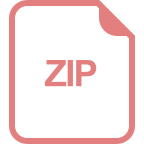
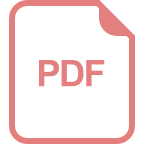
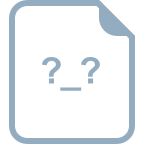
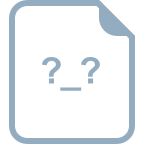
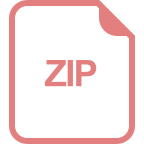
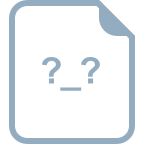
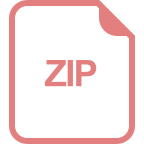



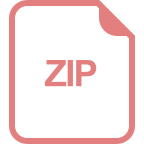
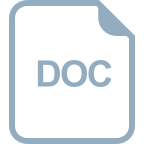
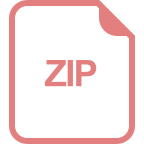