使用idea搭建一个Git项目,技术使用maven、springboot、mybatis plus、redis等
时间: 2024-01-01 17:03:33 浏览: 186
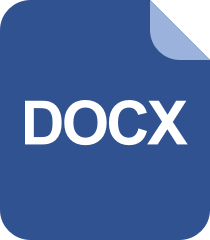
idea+springBoot+maven 搭建项目
1. 首先,在idea中创建一个新的maven项目,选择Spring Initializr模板,添加所需要的依赖:
- Spring Boot DevTools
- Spring Web
- MyBatis Plus
- Redis
2. 在pom.xml中添加如下配置:
```xml
<properties>
<java.version>1.8</java.version>
<mybatis-plus.version>3.4.2</mybatis-plus.version>
<redisson.version>3.16.1</redisson.version>
</properties>
<dependencies>
<!-- Spring Boot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
<!-- MyBatis Plus -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>${mybatis-plus.version}</version>
</dependency>
<!-- Redis -->
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson</artifactId>
<version>${redisson.version}</version>
</dependency>
<!-- Junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
```
3. 在application.yml中添加如下配置:
```yaml
server:
port: 8080
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&useSSL=false&serverTimezone=GMT%2B8
username: root
password: 123456
redis:
host: localhost
port: 6379
database: 0
```
4. 创建一个UserController,实现用户的增删改查功能:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/add")
public boolean addUser(@RequestBody User user) {
return userService.addUser(user);
}
@DeleteMapping("/delete/{id}")
public boolean deleteUser(@PathVariable("id") Long id) {
return userService.deleteUser(id);
}
@PutMapping("/update")
public boolean updateUser(@RequestBody User user) {
return userService.updateUser(user);
}
@GetMapping("/get/{id}")
public User getUserById(@PathVariable("id") Long id) {
return userService.getUserById(id);
}
@GetMapping("/list")
public List<User> getUserList() {
return userService.getUserList();
}
}
```
5. 创建一个User实体类:
```java
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
```
6. 创建一个UserService,定义用户的增删改查接口:
```java
public interface UserService {
boolean addUser(User user);
boolean deleteUser(Long id);
boolean updateUser(User user);
User getUserById(Long id);
List<User> getUserList();
}
```
7. 实现UserService接口,在其中使用MyBatis Plus操作数据库:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public boolean addUser(User user) {
return userMapper.insert(user) > 0;
}
@Override
public boolean deleteUser(Long id) {
return userMapper.deleteById(id) > 0;
}
@Override
public boolean updateUser(User user) {
return userMapper.updateById(user) > 0;
}
@Override
public User getUserById(Long id) {
return userMapper.selectById(id);
}
@Override
public List<User> getUserList() {
return userMapper.selectList(null);
}
}
```
8. 创建一个UserMapper接口,继承BaseMapper<User>,使用MyBatis Plus操作数据库:
```java
@Mapper
@Repository
public interface UserMapper extends BaseMapper<User> {
}
```
9. 在启动类中添加@EnableCaching注解,开启缓存:
```java
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
10. 在UserService中使用Redis缓存,优化数据库操作:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Autowired
private RedissonClient redissonClient;
@Override
@Cacheable(value = "userCache", key = "#user.id")
public boolean addUser(User user) {
return userMapper.insert(user) > 0;
}
@Override
@CacheEvict(value = "userCache", key = "#id")
public boolean deleteUser(Long id) {
return userMapper.deleteById(id) > 0;
}
@Override
@CachePut(value = "userCache", key = "#user.id")
public boolean updateUser(User user) {
return userMapper.updateById(user) > 0;
}
@Override
@Cacheable(value = "userCache", key = "#id")
public User getUserById(Long id) {
RBucket<User> userBucket = redissonClient.getBucket("user:" + id);
User user = userBucket.get();
if (user == null) {
user = userMapper.selectById(id);
userBucket.set(user);
}
return user;
}
@Override
@Cacheable(value = "userCache")
public List<User> getUserList() {
return userMapper.selectList(null);
}
}
```
以上就是使用idea搭建一个Git项目,技术使用maven、springboot、mybatis plus、redis等的详细步骤。
阅读全文
相关推荐
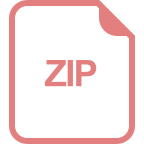
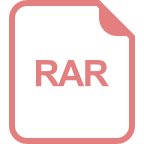

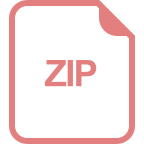
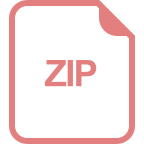
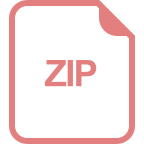
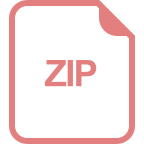
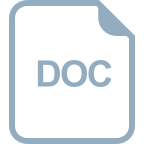
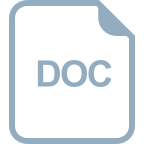
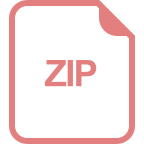
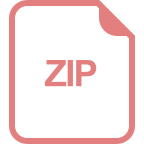
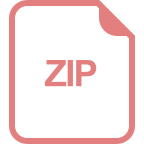
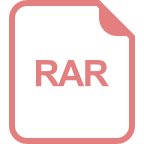
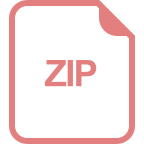
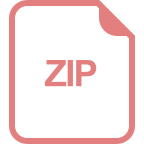
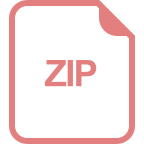
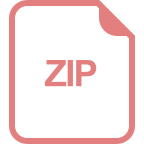
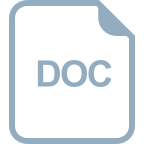