有趣的python代码
时间: 2023-07-10 10:04:48 浏览: 73
以下是一些有趣的 Python 代码示例:
1. 打印一个彩色的“Hello World!”:
```python
print("\033[1;31;40mHello World!\033[0m")
```
2. 生成一个随机密码:
```python
import random
import string
def generate_password(length):
letters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(random.choice(letters) for i in range(length))
return password
print(generate_password(16))
```
3. 用 Turtle 绘制一个彩色的螺旋线:
```python
from turtle import *
speed(0)
bgcolor('black')
colors = ['red', 'purple', 'blue', 'green', 'yellow', 'orange']
for x in range(360):
pencolor(colors[x % 6])
width(x / 100 + 1)
forward(x)
left(59)
```
4. 使用 Pygame 播放一首 MP3 音乐:
```python
import pygame
pygame.init()
pygame.mixer.music.load('music.mp3')
pygame.mixer.music.set_volume(0.7)
pygame.mixer.music.play()
while pygame.mixer.music.get_busy():
pygame.time.Clock().tick(10)
```
5. 使用 Pillow 库生成一个 ASCII 艺术:
```python
from PIL import Image
ASCII_CHARS = ['@', '#', 'S', '%', '?', '*', '+', ';', ':', ',', '.']
def resize_image(image, new_width=100):
width, height = image.size
ratio = height / width
new_height = int(new_width * ratio)
resized_image = image.resize((new_width, new_height))
return resized_image
def grayify(image):
grayscale_image = image.convert('L')
return grayscale_image
def pixels_to_ascii(image):
pixels = image.getdata()
characters = ''.join([ASCII_CHARS[pixel // 25] for pixel in pixels])
return characters
def main():
image_path = 'image.jpg'
new_width = 100
image = Image.open(image_path)
image = resize_image(image, new_width=new_width)
image = grayify(image)
ascii_pixels = pixels_to_ascii(image)
len_pixels = len(ascii_pixels)
ascii_image = '\n'.join([ascii_pixels[index:(index+new_width)] for index in range(0, len_pixels, new_width)])
print(ascii_image)
main()
```
这些代码只是一些示例,希望能够给你带来灵感和启发。
阅读全文
相关推荐
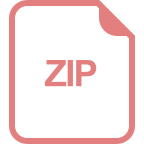
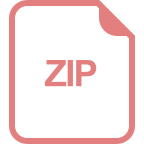
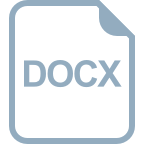
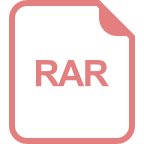
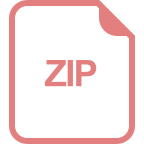
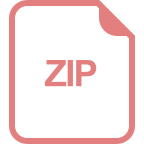
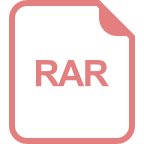
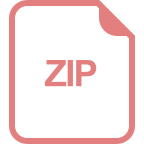
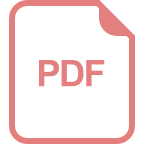
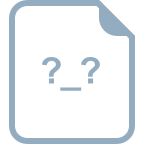
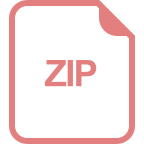
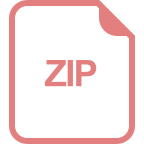
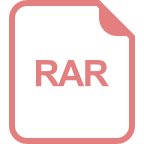
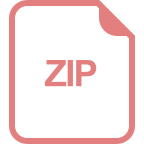
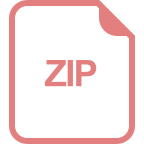
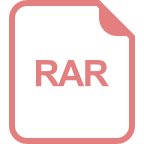