springboot kryo
时间: 2024-12-26 18:25:01 浏览: 6
### Spring Boot 集成 Kryo 序列化
为了在 Spring Boot 中集成 Kryo 进行对象序列化,可以按照如下方法配置:
#### 添加依赖项
首先,在 `pom.xml` 文件中添加必要的 Maven 依赖来引入 Kryo 和其他支持库。
```xml
<dependency>
<groupId>com.esotericsoftware</groupId>
<artifactId>kryo</artifactId>
<version>5.0.0</version>
</dependency>
<!-- 如果需要使用反射特性 -->
<dependency>
<groupId>com.esotericsoftware</groupId>
<artifactId>minlog</artifactId>
<version>1.3.0</version>
</dependency>
```
#### 创建自定义的 Serializer Bean
接着创建一个类用于注册并返回已初始化好的 Kryo 实例作为 bean 注入到 Spring 上下文中。
```java
import com.esotericsoftware.kryo.Kryo;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class KryoConfig {
@Bean(name = "kryoSerializer")
public Kryo getKryo() {
final Kryo kryo = new Kryo();
// Register classes that will be serialized here.
// For example:
// kryo.register(MyClass.class);
return kryo;
}
}
```
#### 使用 Kryo 对象进行序列化/反序列化操作
当有了上述配置之后就可以通过注入的方式获取这个 Kryo 的实例来进行数据转换工作了。下面是一个简单的例子展示怎样利用它完成基本的对象保存读取功能[^1]。
```java
@Autowired
private Kryo kryo;
// Serialization method
public byte[] serialize(Object obj) throws Exception {
Output output = null;
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
output = new Output(baos);
kryo.writeClassAndObject(output, obj);
return output.toBytes();
} finally {
if (output != null && !output.closed)
output.close();
}
}
// Deserialization method
@SuppressWarnings("unchecked")
public <T> T deserialize(byte[] bytes) throws Exception {
Input input = null;
try (ByteArrayInputStream bais = new ByteArrayInputStream(bytes)) {
input = new Input(bais);
return (T) kryo.readClassAndObject(input);
} finally {
if (input != null && !input.eof())
input.close();
}
}
```
以上就是关于如何在一个典型的 Spring Boot 应用程序里边加入对 Kryo 支持的一个简单教程。需要注意的是实际开发过程中可能还需要考虑线程安全等问题以及针对特定业务场景做适当调整优化。
阅读全文
相关推荐
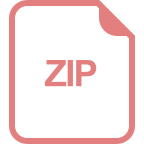
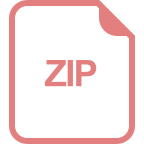
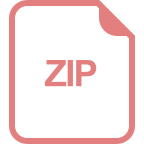
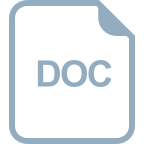






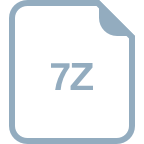
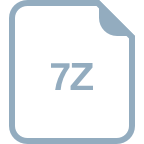
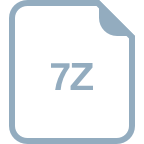
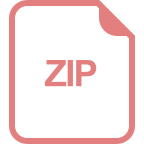
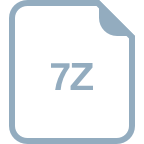
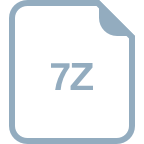
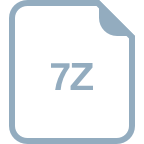
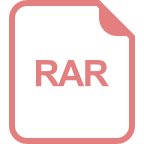
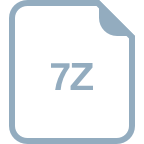
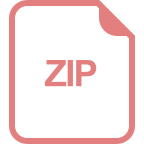