串口缓冲区代码
时间: 2023-11-03 10:05:36 浏览: 78
下面是一个简单的串口接收缓冲区代码示例,使用单字节缓冲区和循环队列实现:
```c
#define BUFFER_SIZE 64
volatile uint8_t rx_buffer[BUFFER_SIZE];
volatile uint8_t rx_head = 0;
volatile uint8_t rx_tail = 0;
// 串口接收中断处理函数
ISR(USART_RX_vect)
{
uint8_t data = UDR0;
uint8_t next_head = (rx_head + 1) % BUFFER_SIZE;
if (next_head != rx_tail) {
rx_buffer[rx_head] = data;
rx_head = next_head;
}
}
// 从接收缓冲区读取一个字节
uint8_t read_byte(void)
{
uint8_t data = 0;
if (rx_head != rx_tail) {
data = rx_buffer[rx_tail];
rx_tail = (rx_tail + 1) % BUFFER_SIZE;
}
return data;
}
// 从接收缓冲区读取指定长度的数据
void read_data(uint8_t *buf, uint8_t len)
{
uint8_t i;
for (i = 0; i < len; i++) {
buf[i] = read_byte();
}
}
```
上述代码中,使用一个大小为 BUFFER_SIZE 的静态数组作为接收缓冲区,rx_head 和 rx_tail 分别表示缓冲区的头和尾指针位置。在串口接收中断处理函数中,将接收到的数据存储到缓冲区中,并更新头指针位置。read_byte 函数用于从缓冲区中读取一个字节的数据,read_data 函数用于从缓冲区中读取指定长度的数据。使用循环队列实现缓冲区,可以有效地避免数据溢出和浪费内存。
阅读全文
相关推荐
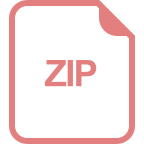
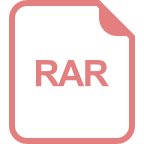
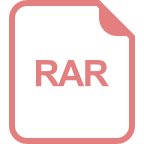
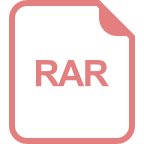
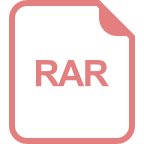
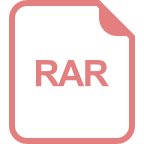
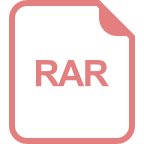
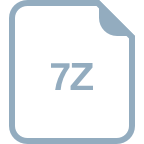
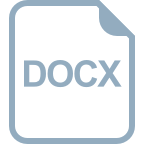

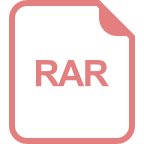
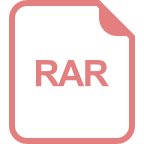
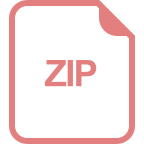
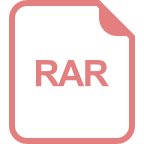
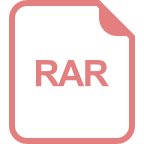
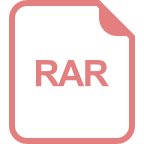
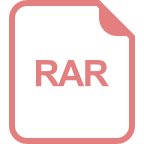