vue退出清空路由缓存
时间: 2023-08-17 10:09:42 浏览: 280
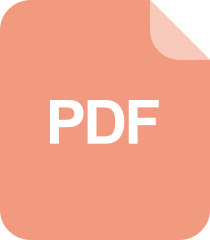
vue spa应用中的路由缓存问题与解决方案
在 Vue 中,可以通过以下几种方式来清空路由缓存并退出:
1. 使用 `router.go(0)` 方法:这个方法会重新加载当前路由,并清空缓存。
```javascript
this.$router.go(0);
```
2. 使用 `router.replace` 方法跳转到一个新的页面,然后再返回原页面:这个方法会替换当前路由,并重新加载页面,从而清空缓存。
```javascript
this.$router.replace('/new-page');
this.$router.replace('/original-page');
```
3. 使用 `router.push` 方法跳转到一个新的页面,然后再返回原页面:这个方法会添加一个新的历史记录,并重新加载页面,从而清空缓存。
```javascript
this.$router.push('/new-page');
this.$router.push('/original-page');
```
请注意,以上方法适用于 Vue Router 2.x 版本。如果你使用的是 Vue Router 3.x 版本,请将 `this.$router` 替换为 `this.$route`。另外,如果你使用了缓存插件(如 `vue-router-cache`),可能需要根据插件的文档来清空缓存。
阅读全文
相关推荐
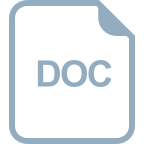
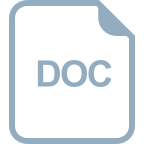
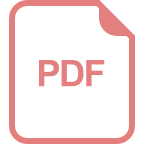


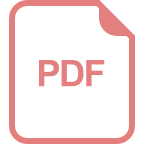
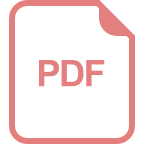
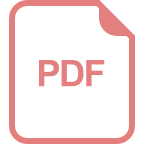
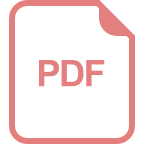
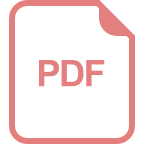
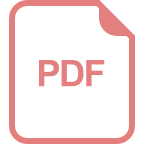
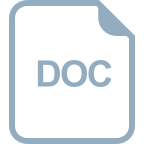





