建立一个简单的学校人员管理系统,包括对学生、员工和在职学生(既是员工又是学生)的管理。需要完成如下功能: 1、建立一个School类,在其中定义增加人员的Append函数。 2、建立一个基类Person类,要具有姓名和性别的属性,并把输出函数ShowMe()定义为虚函数; 3、建立一个员工Staff类和一个学生类Student,均由Person继承而来。要求可以输出员工类(学生类)对象的属性(姓名、性别和工作证号码(或学生学号),分别写出对ShowMe()函数的具体实现。 4、建立一个在职学生类Staff_Student类,由员工类和学生类继承而来。写出对ShowMe()函数的具体实现,可以输出对象属性,。 5、重载,实现用cin为员工类、学生类和在职学生类对象赋值。 6、编写main()主函数,测试上述功能。
时间: 2024-03-04 22:47:49 浏览: 165
好的,我会根据您的要求为您设计一个学校人员管理系统,以下是代码实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Person {
private:
string name;
string gender;
public:
Person(string n, string g) : name(n), gender(g) {}
virtual void ShowMe() {
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
}
};
class Staff : public Person {
private:
string work_id;
public:
Staff(string n, string g, string w) : Person(n, g), work_id(w) {}
void ShowMe() {
Person::ShowMe();
cout << "Work ID: " << work_id << endl;
}
friend istream& operator>>(istream& in, Staff& s) {
cout << "Please input staff's name:";
in >> s.name;
cout << "Please input staff's gender:";
in >> s.gender;
cout << "Please input staff's work ID:";
in >> s.work_id;
return in;
}
};
class Student : public Person {
private:
string stu_id;
public:
Student(string n, string g, string s) : Person(n, g), stu_id(s) {}
void ShowMe() {
Person::ShowMe();
cout << "Student ID: " << stu_id << endl;
}
friend istream& operator>>(istream& in, Student& s) {
cout << "Please input student's name:";
in >> s.name;
cout << "Please input student's gender:";
in >> s.gender;
cout << "Please input student's student ID:";
in >> s.stu_id;
return in;
}
};
class Staff_Student : public Staff, public Student {
public:
Staff_Student(string n, string g, string w, string s) : Staff(n, g, w), Student(n, g, s) {}
void ShowMe() {
Person::ShowMe();
cout << "Work ID: " << Staff::work_id << endl;
cout << "Student ID: " << Student::stu_id << endl;
}
friend istream& operator>>(istream& in, Staff_Student& ss) {
cout << "Please input staff-student's name:";
in >> ss.name;
cout << "Please input staff-student's gender:";
in >> ss.gender;
cout << "Please input staff-student's work ID:";
in >> ss.work_id;
cout << "Please input staff-student's student ID:";
in >> ss.stu_id;
return in;
}
};
class School {
private:
vector<Person*> people;
public:
void Append(Person* p) {
people.push_back(p);
}
void ShowAll() {
for (auto p : people) {
p->ShowMe();
}
}
};
int main() {
School school;
int n;
cout << "Please input the number of staffs, students and staff-students:";
cin >> n;
for (int i = 1; i <= n; i++) {
int type;
cout << "Please input the type of person (1 for staff, 2 for student, 3 for staff-student):";
cin >> type;
switch (type) {
case 1: {
auto s = new Staff("", "", "");
cin >> *s;
school.Append(s);
break;
}
case 2: {
auto s = new Student("", "", "");
cin >> *s;
school.Append(s);
break;
}
case 3: {
auto s = new Staff_Student("", "", "", "");
cin >> *s;
school.Append(s);
break;
}
}
}
school.ShowAll();
return 0;
}
```
这个程序实现了一个学校人员管理系统,可以添加员工、学生和在职学生,并可以输出这些人员的属性。同时,程序中还重载了输入运算符,可以通过cin为对象赋值。运行程序后,会先要求输入需要添加的员工、学生和在职学生的数量,然后逐个输入每个人员的信息,最后输出所有人员的属性。
阅读全文
相关推荐
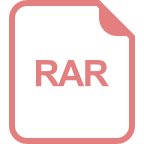
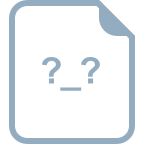
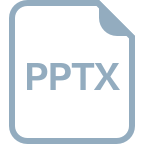
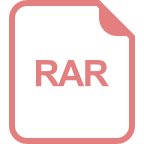
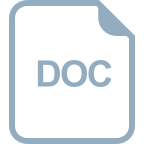
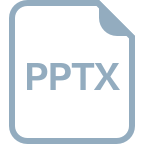
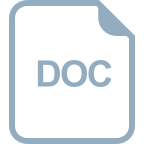
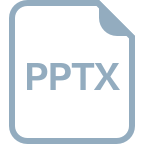
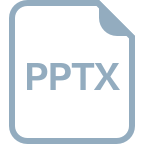
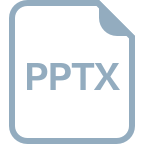
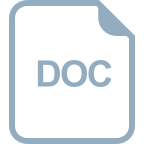

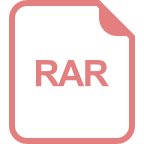
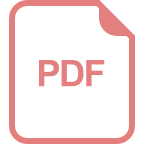
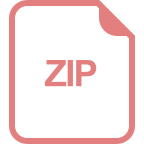