51单片机读取DHT11温湿度传感器代码
时间: 2023-08-28 15:04:09 浏览: 215
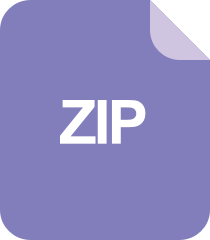
51单片机&DHT11传感器&ESP8266模块实现无线测控温湿度原理图+BOM+软件源码.zip

### 回答1:
好的,下面是 51 单片机读取 DHT11 温湿度传感器的代码示例:
#include <reg51.h>
#include <intrins.h>
sbit DHT11_Data = P1^0;
unsigned char DHT11_Rx_Byte()
{
unsigned char i, dat;
dat = 0;
for (i=0; i<8; i++)
{
while(!DHT11_Data);
_nop_();
_nop_();
dat <<= 1;
if(DHT11_Data)
{
dat |= 1;
}
while(DHT11_Data);
}
return dat;
}
void main()
{
unsigned char humi_int, humi_deci, temp_int, temp_deci, check;
while(1)
{
DHT11_Data = 1;
_nop_();
_nop_();
DHT11_Data = 0;
_nop_();
_nop_();
DHT11_Data = 1;
while(!DHT11_Data);
while(DHT11_Data);
humi_int = DHT11_Rx_Byte();
humi_deci = DHT11_Rx_Byte();
temp_int = DHT11_Rx_Byte();
temp_deci = DHT11_Rx_Byte();
check = DHT11_Rx_Byte();
if((humi_int + humi_deci + temp_int + temp_deci) == check)
{
// 数据校验正确,可以使用 humi_int、humi_deci、temp_int 和 temp_deci 变量中的数据
}
else
{
// 数据校验错误,不能使用 humi_int、humi_deci、temp_int 和 temp_deci 变量中的数据
}
}
}
该代码实现了 51 单片机读取 DHT11 温湿度传感器的功能。它首先向 DHT11 发送信号,然后通过调用 DHT11_Rx_Byte 函数接收 DHT11 发送回来的数据。最后,它还进行了数据校验,确保数据的准确性。
希望这能帮
### 回答2:
以下是51单片机读取DHT11温湿度传感器的示例代码:
#include <reg51.h>
sbit DHT11_PIN = P1^0; // 定义DHT11信号引脚
void delay_us(unsigned int t) // 微秒级延时函数
{
while (t--)
{
// 通过经验值估算延时时间
for(int i=0; i<11; i++);
}
}
void delay_ms(unsigned int t) // 毫秒级延时函数
{
while (t--)
{
delay_us(1000);
}
}
void start_signal() // 发送起始信号
{
DHT11_PIN = 0;
delay_ms(20);
DHT11_PIN = 1;
delay_us(30);
}
unsigned char read_byte() // 读取一个字节的数据
{
unsigned char byte = 0;
for (int i = 0; i < 8; i++)
{
while(!DHT11_PIN); // 等待高电平信号过去
delay_us(30);
if(DHT11_PIN)
{
byte |= (1 << (7 - i)); // 高位在前,低位在后
}
while(DHT11_PIN); // 等待低电平信号过去
}
return byte;
}
void main()
{
unsigned char temperature, humidity;
while(1)
{
start_signal();
while(!DHT11_PIN); // 等待DHT11的响应信号
while(DHT11_PIN); // 等待DHT11的响应信号过去
if(DHT11_PIN)
{
temperature = read_byte(); // 读取温度值的整数部分
read_byte(); // 读取温度值的小数部分
humidity = read_byte(); // 读取湿度值的整数部分
read_byte(); // 读取湿度值的小数部分
// 打印温湿度值
// ...
// 进行其他操作
// ...
}
delay_ms(2000); // 2秒钟后重新读取温湿度值
}
}
以上代码是使用51单片机读取DHT11温湿度传感器数据的一个示例。其中,通过定义DHT11_PIN为P1^0来指定DHT11信号引脚。通过起始信号和读取字节的函数来与DHT11进行通信。最后,通过打印温湿度值或进行其他操作来处理传感器数据。整个过程会附带一些延时函数来确保正确的通信和操作时序。
### 回答3:
51单片机读取DHT11温湿度传感器的代码如下:
#include <reg51.h>
sbit DHT11_DATA = P1^0;
void delay_us(unsigned int t)
{
while(t--)
{
_nop_();
}
}
unsigned char read_byte()
{
unsigned char i, dat;
for(i = 0; i < 8; i++)
{
while(!DHT11_DATA);
delay_us(30);
if(DHT11_DATA)
{
dat |= (1 << (7 - i));
while(DHT11_DATA);
}
else
{
while(!DHT11_DATA);
}
}
return dat;
}
void read_DHT11(unsigned int *temp, unsigned int *humi)
{
unsigned char i, dat[5];
DHT11_DATA = 0;
delay_us(20000);
DHT11_DATA = 1;
delay_us(30);
if(!DHT11_DATA)
{
while(!DHT11_DATA);
while(DHT11_DATA);
for(i = 0; i < 5; i++)
{
dat[i] = read_byte();
}
if(dat[0] + dat[1] + dat[2] + dat[3] == dat[4])
{
*humi = dat[0];
*temp = dat[2];
}
}
}
void main()
{
unsigned int temperature, humidity;
read_DHT11(&temperature, &humidity);
// 处理温湿度数据
while(1);
}
以上代码首先定义了51单片机的引脚和一些延时函数。read_byte()函数用来读取DHT11传感器发送的数据,根据数据位的高低电平判断是0还是1,并将数据保存在变量dat中。read_DHT11()函数用来读取DHT11传感器的温湿度数据,并将其保存在变量temp和humi中。主函数调用read_DHT11()函数获取温湿度数据,并进行后续处理。
请注意,以上代码仅供参考,具体的温湿度传感器型号和引脚连接可能会有所不同,请根据实际情况进行相应的修改。
阅读全文
相关推荐
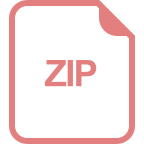
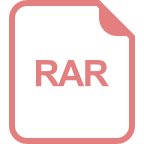
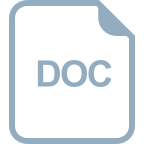
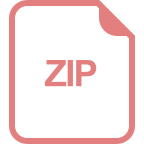
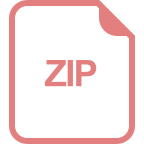
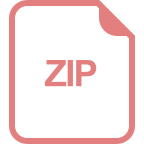
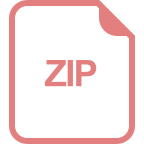
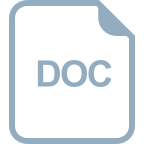
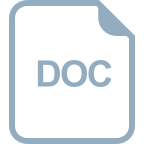
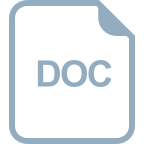
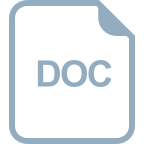
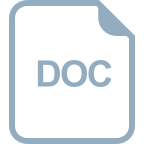
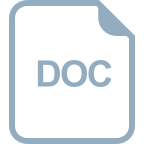
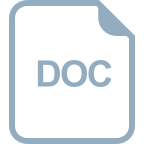
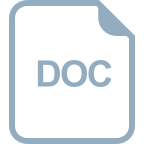