使用python提取json输出为测试报告界面
时间: 2023-07-16 18:15:48 浏览: 46
可以使用Python中的unittest和HTMLTestRunner模块来实现将测试结果输出为HTML格式的测试报告。
首先,需要将测试结果保存为json格式的文件。可以使用Python中的json模块来将测试结果转换为json格式并保存到文件中。
示例代码如下:
```python
import json
# 测试结果字典
result = {
"test_case_1": "pass",
"test_case_2": "fail",
"test_case_3": "pass"
}
# 将测试结果保存为json格式文件
with open("test_result.json", "w") as f:
json.dump(result, f)
```
接下来,可以使用unittest模块来编写测试用例,并将测试结果保存到json文件中。
示例代码如下:
```python
import unittest
import json
class TestMyApp(unittest.TestCase):
def test_case_1(self):
self.assertTrue(True)
def test_case_2(self):
self.assertFalse(False)
def test_case_3(self):
self.assertTrue(True)
if __name__ == '__main__':
# 运行测试用例并将结果保存为json格式文件
with open("test_result.json", "w") as f:
runner = unittest.TextTestRunner(stream=f, verbosity=2)
result = runner.run(unittest.TestLoader().loadTestsFromTestCase(TestMyApp))
json.dump(result.__dict__, f)
```
最后,可以使用HTMLTestRunner模块来将json文件转换为HTML格式的测试报告。
示例代码如下:
```python
import json
import unittest
import HTMLTestRunner
class TestMyApp(unittest.TestCase):
def test_case_1(self):
self.assertTrue(True)
def test_case_2(self):
self.assertFalse(False)
def test_case_3(self):
self.assertTrue(True)
if __name__ == '__main__':
# 运行测试用例并将结果保存为json格式文件
with open("test_result.json", "w") as f:
runner = unittest.TextTestRunner(stream=f, verbosity=2)
result = runner.run(unittest.TestLoader().loadTestsFromTestCase(TestMyApp))
json.dump(result.__dict__, f)
# 将json文件转换为HTML格式测试报告
with open("test_result.json") as f:
result = json.load(f)
with open("test_report.html", "wb") as f:
HTMLTestRunner.HTMLTestRunner(stream=f, verbosity=2).run(result["testsRun"])
```
运行以上代码后,会在当前目录下生成test_result.json和test_report.html两个文件,其中test_report.html即为测试报告界面。
相关推荐
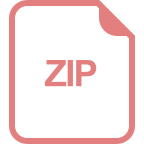
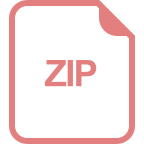
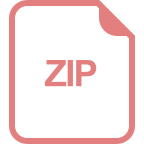
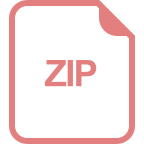
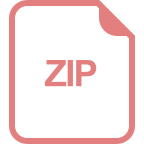
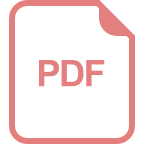
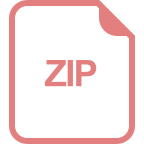
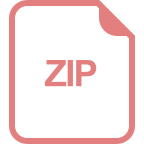
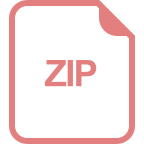
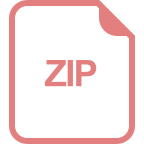
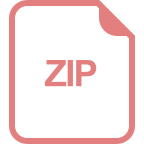
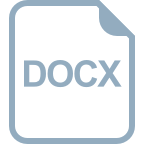
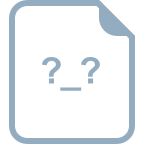
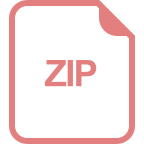
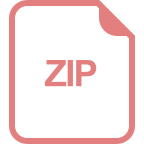
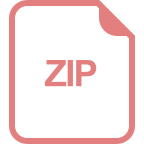
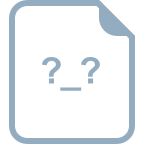
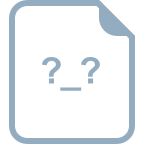