spire.doc在书签位置插入多个表格
时间: 2024-01-01 18:44:58 浏览: 237
可以使用Spire.Doc组件的API来实现在书签位置插入多个表格,具体步骤如下:
1. 打开Word文档并定位到所需的书签位置;
2. 创建表格对象并设置表格的行数和列数;
3. 通过表格对象的Rows属性获取表格的所有行,并循环遍历每一行;
4. 通过表格行的Cells属性获取行中的所有单元格,并循环遍历每一个单元格;
5. 在每一个单元格中添加需要显示的内容;
6. 将表格对象添加到Document对象中;
7. 保存并关闭Word文档。
示例代码如下:
```
using Spire.Doc;
using Spire.Doc.Documents;
namespace InsertMultipleTables
{
class Program
{
static void Main(string[] args)
{
// 打开Word文档
Document document = new Document();
document.LoadFromFile("example.docx");
// 定位到书签位置
BookmarksNavigator navigator = new BookmarksNavigator(document);
navigator.MoveToBookmark("table_bookmark");
// 创建表格对象并设置行列数
Table table1 = new Table(document);
table1.ResetCells(3, 3);
// 循环遍历每一个单元格并添加需要的内容
for (int i = 0; i < 3; i++)
{
TableRow row = table1.Rows[i];
for (int j = 0; j < 3; j++)
{
TableCell cell = row.Cells[j];
Paragraph para = new Paragraph(document);
para.AppendText("行" + i + "列" + j);
cell.AddParagraph(para);
}
}
// 将表格添加到Document对象中
navigator.InsertTable(table1);
// 创建另外一个表格对象并设置行列数
Table table2 = new Table(document);
table2.ResetCells(2, 4);
// 循环遍历每一个单元格并添加需要的内容
for (int i = 0; i < 2; i++)
{
TableRow row = table2.Rows[i];
for (int j = 0; j < 4; j++)
{
TableCell cell = row.Cells[j];
Paragraph para = new Paragraph(document);
para.AppendText("行" + i + "列" + j);
cell.AddParagraph(para);
}
}
// 将第二个表格添加到Document对象中
navigator.InsertTable(table2);
// 保存并关闭Word文档
document.SaveToFile("output.docx", FileFormat.Docx);
document.Close();
}
}
}
```
阅读全文
相关推荐
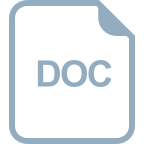
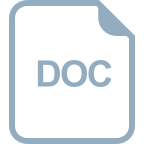
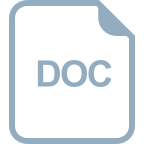
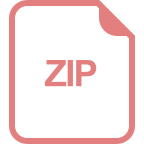
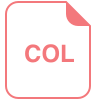
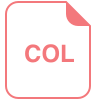

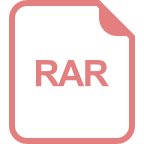
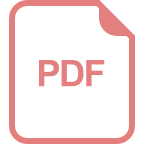
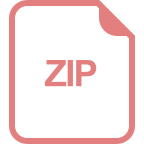
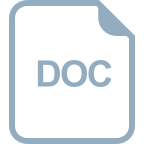
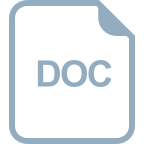
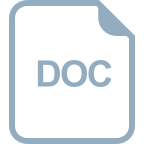
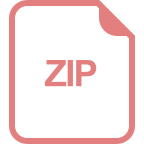
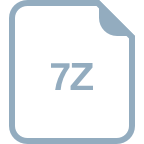
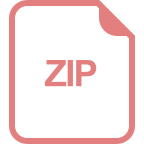
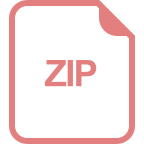
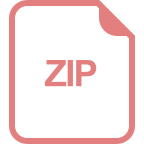