象棋游戏Java代码
时间: 2023-07-03 08:27:13 浏览: 100
好的,以下是一个简单的基于Java Swing实现的象棋游戏代码示例:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ChessGame extends JFrame implements ActionListener {
private JButton[][] board;
private int[][] pieces;
private boolean redTurn;
public ChessGame() {
setTitle("Chinese Chess");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
board = new JButton[10][9];
pieces = new int[][] {
{2, 3, 4, 5, 6, 5, 4, 3, 2},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 7, 0, 0, 0, 0, 0, 7, 0},
{1, 0, 1, 0, 1, 0, 1, 0, 1},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{-1, 0, -1, 0, -1, 0, -1, 0, -1},
{0, -7, 0, 0, 0, 0, 0, -7, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0},
{-2, -3, -4, -5, -6, -5, -4, -3, -2}
};
redTurn = true;
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(10, 9));
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 9; j++) {
board[i][j] = new JButton();
board[i][j].addActionListener(this);
panel.add(board[i][j]);
if (pieces[i][j] != 0) {
board[i][j].setIcon(new ImageIcon(getPieceImage(pieces[i][j])));
}
}
}
getContentPane().add(panel);
setVisible(true);
}
private Image getPieceImage(int piece) {
String filename = "";
switch (Math.abs(piece)) {
case 1: filename = "jiang"; break;
case 2: filename = "shi"; break;
case 3: filename = "xiang"; break;
case 4: filename = "che"; break;
case 5: filename = "ma"; break;
case 6: filename = "pao"; break;
case 7: filename = "bing"; break;
}
if (piece < 0) {
filename += "_r";
} else {
filename += "_b";
}
filename += ".gif";
return new ImageIcon(filename).getImage();
}
public void actionPerformed(ActionEvent e) {
for (int i = 0; i < 10; i++) {
for (int j = 0; j < 9; j++) {
if (e.getSource() == board[i][j]) {
if (pieces[i][j] != 0 && pieces[i][j] < 0 == redTurn) {
// select a piece
} else if (pieces[i][j] == 0) {
// move the selected piece
}
return;
}
}
}
}
public static void main(String[] args) {
new ChessGame();
}
}
```
这段代码使用了一个二维数组来表示棋盘上的棋子,其中正数表示红方棋子,负数表示黑方棋子。例如,1表示红方的“将”,-1表示黑方的“将”。
在界面上,我们使用了一个JPanel和JButton来表示棋盘和棋子。每个按钮都有一个ActionListener,当点击按钮时,会触发actionPerformed方法,我们可以在该方法中处理棋子的选取和移动。
当选取一个棋子时,我们可以通过记录下该棋子的位置,然后在移动时检查是否符合规则(例如“将”只能在九宫格内移动,车只能直线移动等等)。
当移动一个棋子时,我们需要检查目标位置是否可以移动(例如是否有己方棋子挡路),如果可以移动,则将该棋子从原位置移动到目标位置,并切换当前玩家。如果移动后导致对方玩
阅读全文
相关推荐
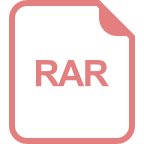
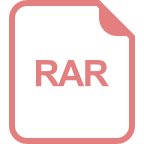
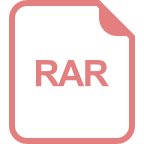
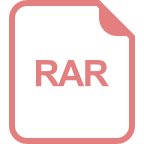
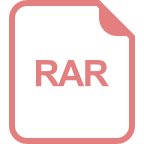
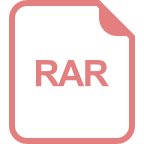
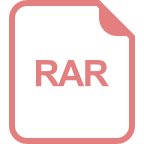
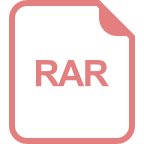
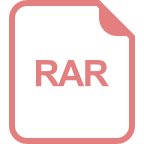
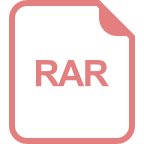
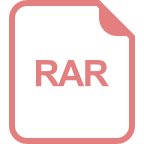
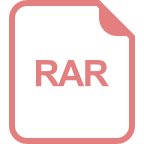
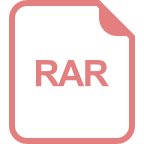
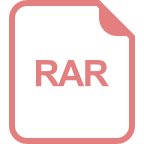
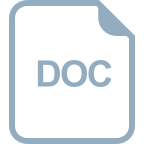
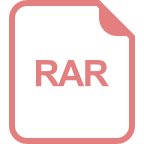
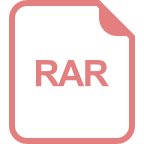
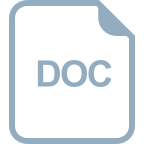
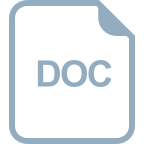