var memoed = MemoInfo.DBCollation.AsQueryable() .Where((t) => { var local = t.LastMemo.ToLocalTime(); return t.UserId == UserInfo.CurrentUser.Id && local < DateTime.Now; })
时间: 2024-02-10 14:30:56 浏览: 26
根据您提供的代码,这是一个LINQ查询表达式,用于从MemoInfo.DBCollation集合中获取当前用户的MemoInfo对象列表,其中最后一条Memo的本地时间早于当前时间。
具体来说,代码使用AsQueryable()方法将MemoInfo.DBCollation集合转换为可查询的IQueryable对象,然后使用Where()方法来筛选符合条件的对象。在Where()方法中,通过lambda表达式定义筛选条件,即UserId等于当前用户的Id,并且最后一条Memo的本地时间早于当前时间。
在lambda表达式中,使用了ToLocalTime()方法将DateTime类型的t.LastMemo转换为本地时间,然后与当前时间DateTime.Now进行比较。最后,查询结果将作为MemoInfo对象列表返回给变量memoed。
相关问题
var memoed = MemoInfo.DBCollation.AsQueryable() .Where((t) => { var local = t.LastMemo.ToLocalTime(); return t.UserId == UserInfo.CurrentUser.Id && local < DateTime.Now; }),语法错误
根据您提供的代码,似乎缺少一个分号(;),该分号应该在代码的末尾。请尝试使用以下代码:
```
var memoed = MemoInfo.DBCollation.AsQueryable()
.Where((t) =>
{
var local = t.LastMemo.ToLocalTime();
return t.UserId == UserInfo.CurrentUser.Id && local < DateTime.Now;
});
```
请注意,除了上述语法错误之外,该代码段本身是有效的,可以从MemoInfo.DBCollation集合中获取符合条件的MemoInfo对象列表,其中最后一条Memo的本地时间早于当前时间。
var Words = WordInfo.DBCollation.AsQueryable().Where(t => t.MemoTime == 0).Take(5).ToList(); BsonArray bsonArray = new BsonArray(); foreach (var model in Words) { bsonArray.Add(model.ToBson()); } // Convert the BsonArray to a byte array byte[] bsonBytes = bsonArray.ToBson();,报错:System.InvalidOperationException:“An Array value cannot be written to the root level of a BSON document.”
The error message indicates that you are trying to write an array value to the root level of a BSON document, which is not allowed.
In your code, you are trying to serialize a `BsonArray` object to a byte array using the `ToBson()` method. However, you cannot serialize an array value to the root level of a BSON document. Instead, you need to wrap the `BsonArray` object in a `BsonDocument` object before serializing it to a byte array.
Here's an updated example code snippet that should work:
```csharp
using MongoDB.Bson;
using MongoDB.Driver;
using System.Collections.Generic;
// Assuming WordInfo class exists with a DBCollation property
List<WordInfo> words = WordInfo.DBCollation.AsQueryable().Where(t => t.MemoTime == 0).Take(5).ToList();
// Serialize the words list to a BsonArray
BsonArray bsonArray = new BsonArray();
foreach (var word in words)
{
bsonArray.Add(word.ToBson());
}
// Wrap the BsonArray in a BsonDocument
BsonDocument bsonDocument = new BsonDocument
{
{ "Words", bsonArray }
};
// Convert the BsonDocument to a byte array
byte[] bsonBytes = bsonDocument.ToBson();
```
In this code snippet, we wrap the `BsonArray` object in a `BsonDocument` object with a key of "Words", and then serialize the `BsonDocument` to a byte array using the `ToBson()` method. This should prevent the "System.InvalidOperationException: An Array value cannot be written to the root level of a BSON document." error.
相关推荐
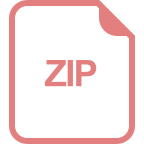
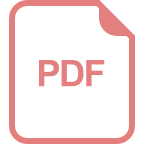
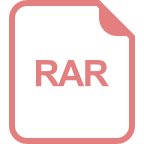













