java解析nc文件工具类
时间: 2024-01-09 18:05:55 浏览: 211
解析 NC 文件需要了解 NC(Numerical Control)码的格式和规则,以及相关的数学知识。如果你不想自己写解析工具类,可以考虑使用第三方库来解析 NC 文件。下面是一些常用的 Java 解析 NC 文件的工具类:
1. JNcParser:JNcParser 是一个 Java 解析 NC 文件的开源库,支持 G 和 M 代码解析,能够读取和解析大部分的 NC 文件。项目地址:https://github.com/BrunoXBSilva/JNcParser
2. NCJava:NCJava 是一个 Java 解析 NC 文件的轻量级库,支持 G 和 M 代码解析,可以读取和解析 NC 文件中的坐标和指令信息。项目地址:https://github.com/edwinm/ncjava
3. JOpenCAM:JOpenCAM 是一个基于 Java 的 CAM 系统,支持读取和解析 NC 文件,生成加工路径和控制机床进行加工。项目地址:https://github.com/psiphi75/jopencam
以上这些工具类都有详细的文档和示例,可以根据自己的需求选择合适的工具类。
相关问题
java解析nc文件工具类代码
以下是一个简单的Java解析nc文件的工具类示例:
```java
import java.io.File;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.IntBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.StandardOpenOption;
public class NcFileParser {
private final static int HEADER_SIZE = 4;
private final static int RECORD_SIZE = 4;
public static void parseNcFile(File file) throws IOException {
try (FileChannel channel = FileChannel.open(file.toPath(), StandardOpenOption.READ)) {
ByteBuffer buffer = ByteBuffer.allocate(HEADER_SIZE).order(ByteOrder.BIG_ENDIAN);
channel.read(buffer);
buffer.rewind();
int header = buffer.getInt();
if (header != 0x43415046) {
throw new IOException("Invalid NetCDF file header");
}
buffer.clear();
channel.read(buffer);
buffer.rewind();
int version = buffer.get();
if (version != 1 && version != 2) {
throw new IOException("Unsupported NetCDF file version");
}
buffer.clear();
channel.read(buffer);
buffer.rewind();
int recordSize = buffer.getInt();
if (recordSize != RECORD_SIZE) {
throw new IOException("Invalid NetCDF header record size");
}
buffer.clear();
channel.position(HEADER_SIZE);
int recordCount = 0;
while (true) {
channel.read(buffer);
buffer.rewind();
int recordID = buffer.getInt();
if (recordID == 0) {
break;
}
int recordLength = buffer.getInt();
buffer.clear();
if (recordID == 1) {
parseDimensionRecord(channel, recordLength);
} else if (recordID == 2) {
parseVariableRecord(channel, recordLength);
} else if (recordID == 3) {
parseAttributeRecord(channel, recordLength);
}
recordCount++;
}
System.out.println("Parsed " + recordCount + " records");
}
}
private static void parseDimensionRecord(FileChannel channel, int recordLength) throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(recordLength).order(ByteOrder.BIG_ENDIAN);
channel.read(buffer);
buffer.rewind();
int dimensionCount = buffer.getInt();
for (int i = 0; i < dimensionCount; i++) {
int dimensionNameLength = buffer.getInt();
byte[] dimensionNameBytes = new byte[dimensionNameLength];
buffer.get(dimensionNameBytes);
String dimensionName = new String(dimensionNameBytes);
int dimensionSize = buffer.getInt();
System.out.println("Dimension: name=" + dimensionName + ", size=" + dimensionSize);
}
}
private static void parseVariableRecord(FileChannel channel, int recordLength) throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(recordLength).order(ByteOrder.BIG_ENDIAN);
channel.read(buffer);
buffer.rewind();
int variableNameLength = buffer.getInt();
byte[] variableNameBytes = new byte[variableNameLength];
buffer.get(variableNameBytes);
String variableName = new String(variableNameBytes);
int variableType = buffer.getInt();
int dimensionCount = buffer.getInt();
int[] dimensionIDs = new int[dimensionCount];
IntBuffer intBuffer = buffer.asIntBuffer();
intBuffer.get(dimensionIDs);
buffer.position(buffer.position() + dimensionCount * 4);
System.out.println("Variable: name=" + variableName + ", type=" + variableType + ", dimensions=" + dimensionIDs);
}
private static void parseAttributeRecord(FileChannel channel, int recordLength) throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(recordLength).order(ByteOrder.BIG_ENDIAN);
channel.read(buffer);
buffer.rewind();
int attributeNameLength = buffer.getInt();
byte[] attributeNameBytes = new byte[attributeNameLength];
buffer.get(attributeNameBytes);
String attributeName = new String(attributeNameBytes);
int attributeType = buffer.getInt();
int attributeLength = buffer.getInt();
if (attributeType == 2) {
byte[] attributeValueBytes = new byte[attributeLength];
buffer.get(attributeValueBytes);
String attributeValue = new String(attributeValueBytes);
System.out.println("Attribute: name=" + attributeName + ", type=" + attributeType + ", value=" + attributeValue);
} else {
System.out.println("Attribute: name=" + attributeName + ", type=" + attributeType + ", length=" + attributeLength);
}
}
}
```
使用示例:
```java
File ncFile = new File("test.nc");
try {
NcFileParser.parseNcFile(ncFile);
} catch (IOException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
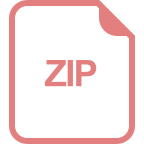
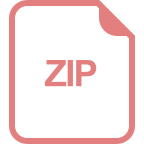
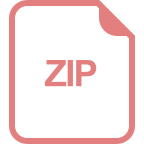
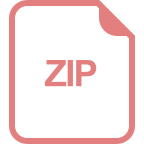
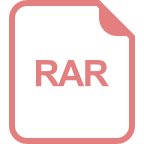
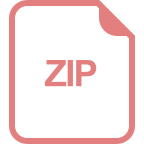
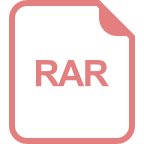
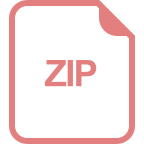
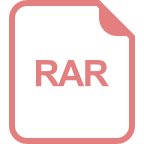
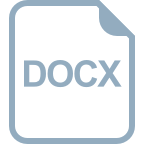
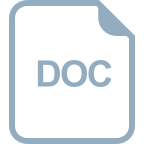
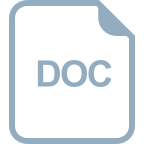
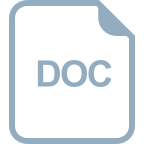
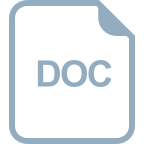
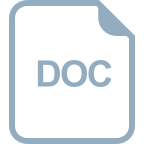