这段代码创建了三个线程,每个线程会随机生成一个目标数字和猜测数字,直到猜中为止,并记录猜的次数。最后,根据猜的次数对线程进行排序,并输出排序结果。 请注意,每次运行结果可能会不同,因为每个线程的猜测是随机的。用java
时间: 2024-04-10 07:27:42 浏览: 40
以下是使用Java编写的多线程猜数字的示例代码:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import java.util.concurrent.ThreadLocalRandom;
class GuessThread extends Thread {
private String name;
private int count;
public GuessThread(String name) {
this.name = name;
this.count = 0;
}
public int getCount() {
return count;
}
@Override
public void run() {
int targetNumber = ThreadLocalRandom.current().nextInt(1, 1001);
int guessNumber = ThreadLocalRandom.current().nextInt(1, 1001);
while (guessNumber != targetNumber) {
count++;
guessNumber = ThreadLocalRandom.current().nextInt(1, 1001);
}
System.out.println(name + " guessed the number " + targetNumber + " in " + count + " tries.");
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
List<GuessThread> threads = new ArrayList<>();
for (int i = 1; i <= 3; i++) {
GuessThread thread = new GuessThread("Thread-" + i);
thread.start();
threads.add(thread);
}
for (GuessThread thread : threads) {
thread.join();
}
threads.sort((t1, t2) -> t1.getCount() - t2.getCount());
System.out.println("Sorted threads based on number of tries:");
for (GuessThread thread : threads) {
System.out.println(thread.getName() + ": " + thread.getCount() + " tries");
}
}
}
```
这段代码使用Java的多线程实现了相同的功能。每个线程会随机生成一个目标数字和猜测数字,直到猜中为止,并记录猜的次数。最后,根据猜的次数对线程进行排序,并输出排序结果。
请注意,每次运行结果可能会不同,因为每个线程的猜测是随机的。
阅读全文
相关推荐
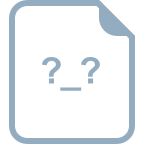
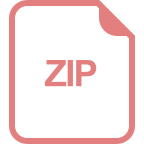
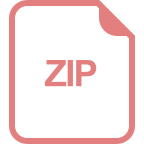
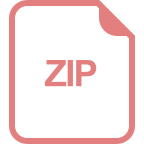
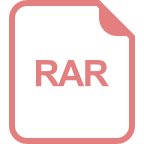
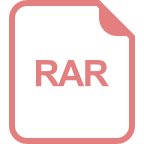
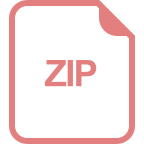
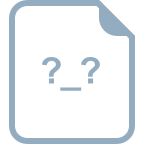
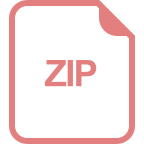
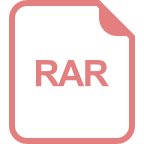
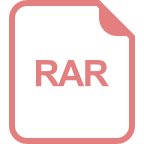
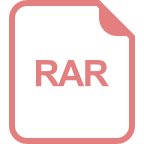
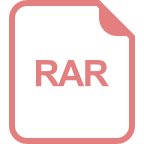
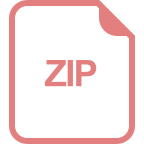
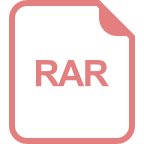
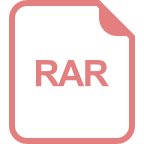