1.绘制peaks两数曲面并且将它绕z轴旋转。 2. 播放一个不断变化的眼球 程序段。 3. 在不同窗口分别绘制三维曲面图、带等高线的曲面图、被光照射带阴影的曲面图、柱面图、球面图等。
时间: 2024-04-28 16:21:32 浏览: 11
好的,我明白了。以下是回答:
1. 绘制peaks两数曲面并且将它绕z轴旋转:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
#生成数据
x = np.arange(-3, 3, 0.1)
y = np.arange(-3, 3, 0.1)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
#绘制曲面图并旋转
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='coolwarm')
for angle in range(0, 360):
ax.view_init(30, angle)
plt.draw()
plt.pause(0.001)
```
2. 播放一个不断变化的眼球程序段:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
#生成数据
x = np.linspace(-1, 1, 100)
y = np.linspace(-1, 1, 100)
X, Y = np.meshgrid(x, y)
Z = np.sqrt(X**2 + Y**2)
#定义update函数
def update(frame):
ax.clear()
ax.plot_surface(X, Y, Z, cmap='coolwarm', alpha=0.5)
ax.scatter(np.sin(frame), np.cos(frame), 0, color='red')
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.set_zlim(0, 2)
ax.set_title('Eye animation')
#绘制动画
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ani = FuncAnimation(fig, update, frames=np.linspace(0, 2*np.pi, 50), interval=50)
plt.show()
```
3. 在不同窗口分别绘制三维曲面图、带等高线的曲面图、被光照射带阴影的曲面图、柱面图、球面图等:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
#生成数据
x = np.linspace(-3, 3, 100)
y = np.linspace(-3, 3, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
#绘制三维曲面图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='coolwarm')
ax.set_title('3D surface plot')
#绘制带等高线的曲面图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='coolwarm')
ax.contour(X, Y, Z, zdir='z', offset=-2, cmap='coolwarm')
ax.set_title('Surface plot with contour')
#绘制被光照射带阴影的曲面图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X, Y, Z, cmap='coolwarm', shade=True)
ax.set_title('Surface plot with shading')
#绘制柱面图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
r = np.linspace(0, 1, 50)
theta = np.linspace(0, 2*np.pi, 50)
R, Theta = np.meshgrid(r, theta)
X = R*np.cos(Theta)
Y = R*np.sin(Theta)
Z = np.sin(X**2 + Y**2)
ax.plot_surface(X, Y, Z, cmap='coolwarm')
ax.set_title('Cylinder plot')
#绘制球面图
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
u = np.linspace(0, 2*np.pi, 50)
v = np.linspace(0, np.pi, 50)
U, V = np.meshgrid(u, v)
X = np.sin(V)*np.cos(U)
Y = np.sin(V)*np.sin(U)
Z = np.cos(V)
ax.plot_surface(X, Y, Z, cmap='coolwarm')
ax.set_title('Sphere plot')
plt.show()
```
相关推荐
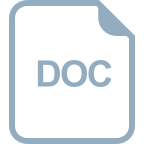
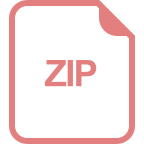














