tkinter中在背景图上绘制一个矩形
时间: 2024-11-15 11:33:34 浏览: 2
在Tkinter中,要在背景图片上绘制一个矩形,你可以通过`PhotoImage`加载图片并将其作为标签的背景,然后利用`create_rectangle`函数在该背景上创建矩形。以下是简单的步骤:
1. 首先,你需要安装PIL(Python Imaging Library)库,用于处理图像。如果你还没有安装,可以使用pip进行安装:
```
pip install pillow
```
2. 然后,在程序中加载图片并转换为`PhotoImage`对象:
```python
from tkinter import *
import tkinter as tk
from PIL import Image, ImageTk
# 加载图片
image_path = "path_to_your_image.jpg"
img = Image.open(image_path)
photo_img = ImageTk.PhotoImage(img)
```
记得替换`"path_to_your_image.jpg"`为你实际的图片路径。
3. 创建一个Tk窗口,并设置背景为图片:
```python
root = tk.Tk()
root.config(bg="white") # 或者将此处的"white"改为`photo_img`,如果想直接用图片做背景
root.title("Background Image with Rectangle")
```
4. 在这个背景上画出矩形:
```python
# 获取图片的宽度和高度
width, height = img.width(), img.height()
# 创建一个矩形的位置和尺寸,这里以图片中心为基准偏移
x = width / 2 - 50
y = height / 2 - 50
rect_width = 100
rect_height = 100
# 使用 PhotoImage 的 create_rectangle 方法在背景上绘图
rectangle = photo_img.create_rectangle(x, y, x + rect_width, y + rect_height, outline="blue", fill="")
```
这里的`outline`和`fill`参数分别控制矩形边框的颜色和填充颜色。你可以根据需要调整它们。
5. 最后,显示窗口:
```python
root.mainloop()
```
阅读全文
相关推荐
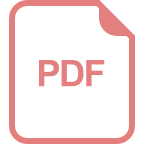
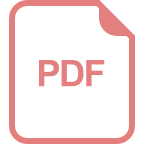
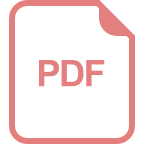



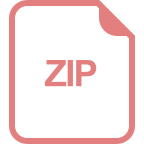
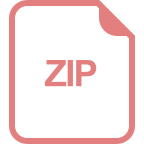
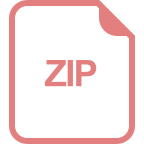
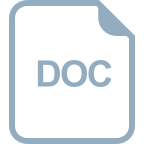
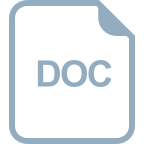
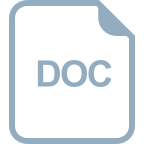
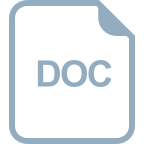
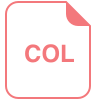




