创建线程类做连续的加法运算,具体:创建一个线程计算1到100的和,另外一个线程计算101到200的和,并把两个线程计算的结果在主线程里相加从而得到1到200的和用Java
时间: 2024-02-28 16:52:04 浏览: 20
好的,下面是一个用Java实现的示例代码,其中使用了Java内置的`Thread`类来创建线程。
```java
class SumThread extends Thread {
private int start, end;
public int result;
public SumThread(int start, int end) {
this.start = start;
this.end = end;
this.result = 0;
}
public void run() {
for (int i = start; i <= end; i++) {
result += i;
}
}
}
public class Main {
public static void main(String[] args) {
// 创建两个线程来计算1到100和101到200的和
SumThread thread1 = new SumThread(1, 100);
SumThread thread2 = new SumThread(101, 200);
// 启动线程
thread1.start();
thread2.start();
try {
// 等待两个线程完成
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// 计算结果
int result = thread1.result + thread2.result;
// 打印结果
System.out.println("1到200的和为:" + result);
}
}
```
在这里,我们创建了一个名为`SumThread`的线程类,它接受两个参数`start`和`end`,表示要计算的和的范围。在`run`方法中,我们使用简单的循环来计算和,并将结果存储在`result`属性中。
在主程序中,我们创建了两个`SumThread`实例来计算两个范围内的和。然后,我们启动这两个线程,并等待它们完成。最后,我们将两个线程的结果相加以得到最终的结果,并将其打印出来。
注意:这个示例代码仅仅是一个简单的例子,实际的多线程编程可能还需要考虑更多的情况,如线程同步、线程安全、异常处理等。
相关推荐
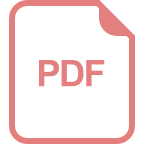














