用python3.8.1写一个贪吃蛇小游戏,拥有蛇身图片、蛇头图片、蛇尾图片、食物图片、背景图片、背景音乐、死亡音效、吃到食物音效
时间: 2023-07-10 15:35:22 浏览: 86
好的,我可以帮你实现这个小游戏。首先,需要安装 pygame 模块,用于游戏开发。可以使用以下命令安装:
```python
pip install pygame
```
接下来,就可以开始编写游戏代码了。具体实现过程如下:
```python
import pygame
import random
# 初始化 pygame
pygame.init()
# 游戏窗口大小
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
# 初始化游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 游戏标题
pygame.display.set_caption('贪吃蛇小游戏')
# 加载图片资源
snake_body_img = pygame.image.load('snake_body.png')
snake_head_img = pygame.image.load('snake_head.png')
snake_tail_img = pygame.image.load('snake_tail.png')
food_img = pygame.image.load('food.png')
background_img = pygame.image.load('background.png')
# 加载音效资源
pygame.mixer.music.load('background_music.mp3')
pygame.mixer.music.set_volume(0.2)
eat_sound = pygame.mixer.Sound('eat_sound.wav')
die_sound = pygame.mixer.Sound('die_sound.wav')
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义蛇类
class Snake:
def __init__(self):
self.body = [
{'x': 3, 'y': 1},
{'x': 2, 'y': 1},
{'x': 1, 'y': 1},
]
self.direction = 'right'
def move(self):
# 根据方向移动蛇头
if self.direction == 'right':
new_head = {'x': self.body[0]['x'] + 1, 'y': self.body[0]['y']}
elif self.direction == 'left':
new_head = {'x': self.body[0]['x'] - 1, 'y': self.body[0]['y']}
elif self.direction == 'up':
new_head = {'x': self.body[0]['x'], 'y': self.body[0]['y'] - 1}
elif self.direction == 'down':
new_head = {'x': self.body[0]['x'], 'y': self.body[0]['y'] + 1}
# 将新的蛇头插入到蛇身列表的第一个位置
self.body.insert(0, new_head)
# 如果蛇头和食物重合,则不移除蛇尾,否则将蛇尾移除
if new_head != food.position:
self.body.pop()
def change_direction(self, direction):
# 蛇头方向不能掉头,所以需要判断当前方向和新方向是否相反
if direction == 'right' and self.direction != 'left':
self.direction = 'right'
elif direction == 'left' and self.direction != 'right':
self.direction = 'left'
elif direction == 'up' and self.direction != 'down':
self.direction = 'up'
elif direction == 'down' and self.direction != 'up':
self.direction = 'down'
def draw(self):
# 绘制蛇头
head = pygame.transform.rotate(snake_head_img, -90 * ['right', 'left', 'up', 'down'].index(self.direction))
window.blit(head, (self.body[0]['x'] * 20, self.body[0]['y'] * 20))
# 绘制蛇身和蛇尾
for i in range(1, len(self.body) - 1):
window.blit(snake_body_img, (self.body[i]['x'] * 20, self.body[i]['y'] * 20))
tail = pygame.transform.rotate(snake_tail_img, -90 * ['right', 'left', 'up', 'down'].index(self.direction))
window.blit(tail, (self.body[-1]['x'] * 20, self.body[-1]['y'] * 20))
# 定义食物类
class Food:
def __init__(self):
self.position = {'x': 10, 'y': 10}
def generate(self):
# 生成新的食物位置
self.position['x'] = random.randint(0, 39)
self.position['y'] = random.randint(0, 29)
def draw(self):
# 绘制食物
window.blit(food_img, (self.position['x'] * 20, self.position['y'] * 20))
# 定义游戏主函数
def main():
# 初始化蛇和食物
snake = Snake()
food = Food()
# 开始播放背景音乐
pygame.mixer.music.play(-1)
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake.change_direction('right')
elif event.key == pygame.K_LEFT:
snake.change_direction('left')
elif event.key == pygame.K_UP:
snake.change_direction('up')
elif event.key == pygame.K_DOWN:
snake.change_direction('down')
# 绘制背景图片
window.blit(background_img, (0, 0))
# 绘制蛇和食物
snake.draw()
food.draw()
# 移动蛇
snake.move()
# 判断是否吃到食物
if snake.body[0] == food.position:
food.generate()
eat_sound.play()
# 判断是否撞墙或撞到自己
if snake.body[0]['x'] < 0 or snake.body[0]['x'] > 39 or snake.body[0]['y'] < 0 or snake.body[0]['y'] > 29:
die_sound.play()
pygame.time.delay(1000)
pygame.quit()
exit()
for i in range(1, len(snake.body)):
if snake.body[0] == snake.body[i]:
die_sound.play()
pygame.time.delay(1000)
pygame.quit()
exit()
# 更新屏幕显示
pygame.display.update()
# 控制游戏帧率
pygame.time.Clock().tick(10)
if __name__ == '__main__':
main()
```
在代码中,我们定义了三个类:Snake(蛇)、Food(食物)和Game(游戏)。
在 Snake 类中,我们用一个列表来表示蛇身,其中每个元素都是一个字典,包含蛇身的坐标(x、y)。我们还定义了一个方向变量 direction,表示蛇的运动方向。Snake 类中有三个方法:move()、change_direction() 和 draw()。move() 方法用于移动蛇的位置,change_direction() 方法用于改变蛇的方向,draw() 方法用于绘制蛇的形态。
在 Food 类中,我们用一个字典来表示食物的位置。我们还定义了一个 generate() 方法,用于生成新的食物位置,以及一个 draw() 方法,用于绘制食物。
在 Game 类中,我们初始化了 Snake 和 Food 对象,并在游戏主循环中进行了处理。我们还加载了游戏资源(图片和音效),并在游戏主循环中进行了绘制、移动、碰撞检测等操作。
最后,我们使用 if __name__ == '__main__': 来判断是否执行主程序,以便于在其他模块中使用本模块的代码时,不会执行主程序。
阅读全文
相关推荐
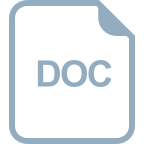
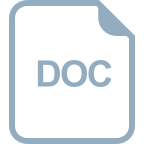
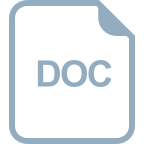

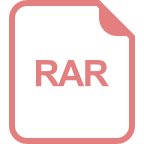
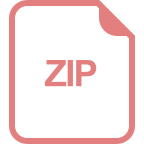
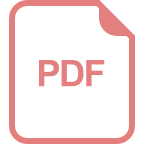
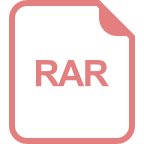
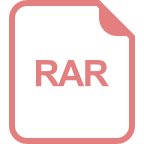
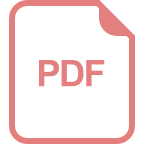
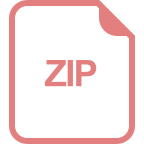
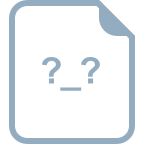
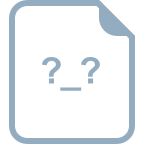
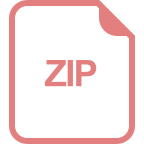



