android 如何使用 ffmpeg 往管道中插入数据
时间: 2024-03-10 15:43:18 浏览: 119
要使用 FFmpeg 将数据插入管道中,您需要使用 FFmpeg 的 API 来编写自定义的应用程序。下面是一个简单的示例代码,演示了如何使用 FFmpeg API 将音频数据写入到命名管道中:
```java
// 初始化 FFmpeg 库
avformat_network_init();
av_register_all();
avcodec_register_all();
// 打开管道
String pipePath = "/data/local/tmp/myfifo";
AVFormatContext formatContext = avformat_alloc_context();
if (avformat_alloc_output_context2(formatContext, null, "fifo", pipePath) < 0) {
return;
}
AVOutputFormat outputFormat = formatContext.oformat;
AVStream outputStream = avformat_new_stream(formatContext, null);
AVCodec codec = avcodec_find_encoder(outputFormat.audio_codec);
AVCodecContext codecContext = avcodec_alloc_context3(codec);
outputStream.codecpar(codecContext);
// 打开编码器
if (avcodec_open2(codecContext, codec, null) < 0) {
return;
}
// 打开管道文件
AVIOContext ioContext = null;
if (avio_open2(ioContext, pipePath, AVIO_FLAG_WRITE, null, null) < 0) {
return;
}
// 创建音频帧
AVFrame frame = av_frame_alloc();
frame.format(codecContext.sample_fmt());
frame.nb_samples(codecContext.frame_size());
frame.channels(codecContext.channels());
// 填充音频数据
byte[] audioData = ...; // 假设这里有音频数据需要写入管道
frame.data(0, audioData);
// 将音频帧写入管道
AVPacket packet = av_packet_alloc();
av_init_packet(packet);
int ret = avcodec_send_frame(codecContext, frame);
if (ret >= 0) {
ret = avcodec_receive_packet(codecContext, packet);
if (ret >= 0) {
packet.stream_index(outputStream.index());
av_packet_rescale_ts(packet, codecContext.time_base(), outputStream.time_base());
av_interleaved_write_frame(formatContext, packet);
}
}
```
这段代码打开了一个名为“/data/local/tmp/myfifo”的命名管道,并使用 FFmpeg API 将音频数据写入管道中。您需要将音频数据填充到 AVFrame 对象中,并使用 avcodec_send_frame() 方法将其发送到编码器。然后使用 avcodec_receive_packet() 方法接收编码后的音频数据,并将其写入管道中。
请注意,这个示例代码仅用于演示 FFmpeg API 的用法。在实际应用中,您需要根据自己的需求进行适当的修改和调整。
阅读全文
相关推荐
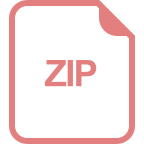
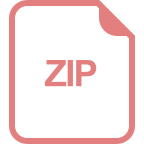
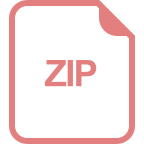
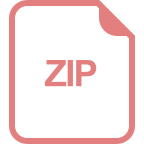
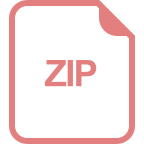
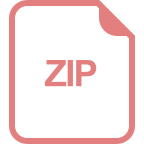
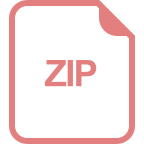
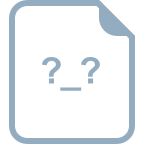
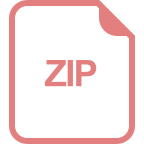
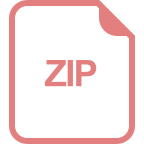
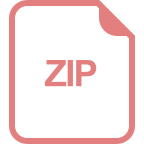
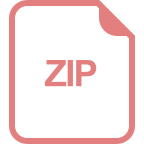
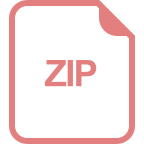
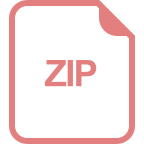
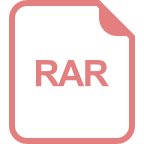