用c++编写:定义有向图用分支界限算法求tsp最优解
时间: 2024-04-08 07:29:52 浏览: 96
下面是使用C++编写的分支限界算法来解决Traveling Salesman Problem(TSP)的最优解:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <limits>
using namespace std;
const int INF = numeric_limits<int>::max();
// 有向图的表示
class Graph {
public:
int vertices;
vector<vector<int>> adjacencyMatrix;
Graph(int v) : vertices(v) {
adjacencyMatrix.resize(v, vector<int>(v, 0));
}
void addEdge(int source, int destination, int weight) {
adjacencyMatrix[source][destination] = weight;
}
};
// 分支限界算法求解TSP
class TSP {
public:
int solve(Graph& graph) {
vector<int> path;
int optimalPath = INF;
vector<bool> visited(graph.vertices, false);
path.push_back(0); // 从节点0开始
solveUtil(graph, path, visited, 0, optimalPath);
return optimalPath;
}
private:
void solveUtil(Graph& graph, vector<int>& path, vector<bool>& visited, int cost, int& optimalPath) {
if (path.size() == graph.vertices) {
// 更新最优路径
optimalPath = min(optimalPath, cost + graph.adjacencyMatrix[path.back()][0]);
return;
}
int last = path.back();
for (int i = 0; i < graph.vertices; i++) {
if (!visited[i]) {
visited[i] = true;
path.push_back(i);
// 计算当前路径的代价
int currentCost = cost + graph.adjacencyMatrix[last][i];
// 剪枝,如果当前路径代价已经大于最优路径,则不再继续搜索
if (currentCost < optimalPath) {
solveUtil(graph, path, visited, currentCost, optimalPath);
}
path.pop_back();
visited[i] = false;
}
}
}
};
int main() {
int vertices, edges;
cout << "Enter the number of vertices: ";
cin >> vertices;
cout << "Enter the number of edges: ";
cin >> edges;
Graph graph(vertices);
cout << "Enter the edges (source, destination, weight):" << endl;
for (int i = 0; i < edges; i++) {
int source, destination, weight;
cin >> source >> destination >> weight;
graph.addEdge(source, destination, weight);
}
TSP tsp;
int optimalPath = tsp.solve(graph);
cout << "Optimal TSP path length: " << optimalPath << endl;
return 0;
}
```
这段代码实现了使用分支限界算法求解TSP的最优路径。你可以输入图的顶点数和边数,然后依次输入每条边的起点、终点和权重。程序将输出最优TSP路径的长度。请确保输入的图是有向图,并且权重都是非负整数。
希望这能帮到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐
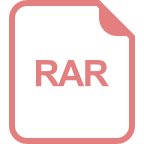

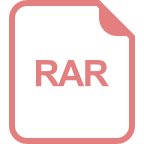
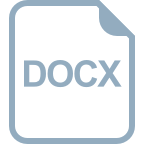
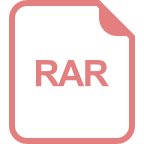
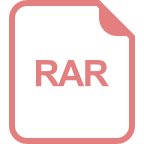
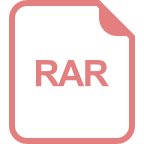
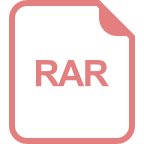
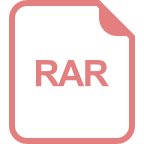
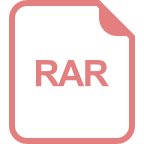
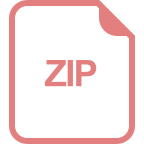
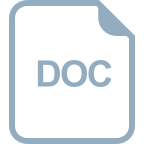
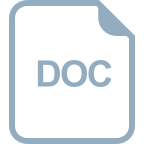