如何在spring boot中访问post请求的网站
时间: 2024-09-18 15:04:28 浏览: 22
在Spring Boot中,要发送POST请求访问另一个网站,你可以使用`RestTemplate`或者第三方库如`HttpURLConnection`, `HttpClient`或者更现代化的`Retrofit`或`OkHttp`。这里我将以`RestTemplate`为例,因为它是最基础的HTTP客户端工具之一:
首先,你需要添加依赖。如果你使用的是Maven,可以在pom.xml文件中添加Spring Web的RestTemplate依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
然后,在你的服务类中创建一个方法来发送POST请求:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Component;
import org.springframework.web.client.RestTemplate;
@Component
public class ExternalApiClient {
private final RestTemplate restTemplate;
@Autowired
public ExternalApiClient(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public String sendPostRequest(String targetUrl, Object requestBody) {
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON); // 根据请求头设置正确的媒体类型
ResponseEntity<String> response = restTemplate.postForEntity(targetUrl, requestBody, String.class);
// 检查响应状态码
response.getStatusCode(); // 如果有权限控制,可以在此检查是否允许访问
return response.getBody(); // 返回接收到的服务器响应
}
}
```
在这个例子中,`targetUrl`是你想要访问的外部网站的URL,`requestBody`是你要发送的POST请求体。注意,`requestBody`的类型应根据实际需要设置,比如JSON、XML或其他数据格式。
要使用这个方法,只需传入URL和合适的请求体,例如:
```java
String apiUrl = "http://example.com/api endpoint";
// 对象实例化,假设是个Map
Map<String, Object> requestData = ...;
String response = externalApiClient.sendPostRequest(apiUrl, requestData);
```
相关推荐
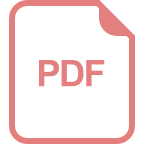
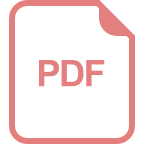
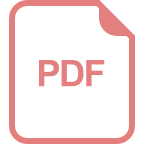
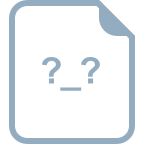
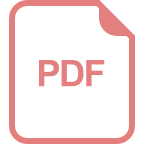
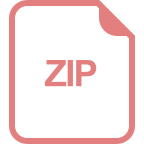
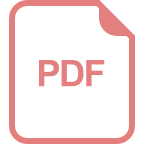
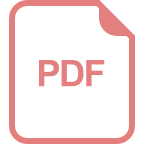
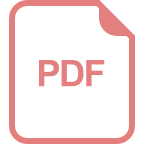
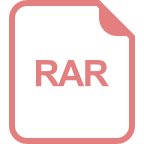
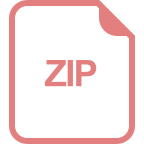
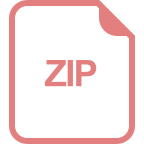
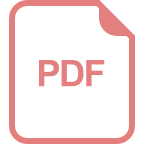
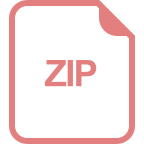
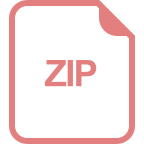
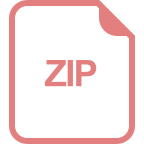
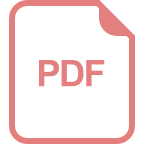
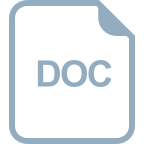
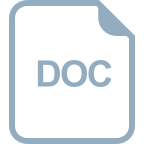