java从其他文件统计字符串个数和非空格字符个数,并存入另一个文件夹
时间: 2024-09-19 18:17:23 浏览: 40
在Java中,你可以通过读取文件、处理内容并写入新文件的方式来完成这个任务。这里是一个简单的步骤说明:
1. 首先,你需要导入`java.io`包,它包含了处理I/O操作的类。
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
```
2. 创建一个方法来读取源文件并计算字符串和非空格字符的数量:
```java
public static void countChars(String inputFile, String outputFile) {
int stringCount = 0;
int nonSpaceCharCount = 0;
try (BufferedReader reader = new BufferedReader(new FileReader(inputFile))) {
String line;
while ((line = reader.readLine()) != null) {
stringCount += line.split("\\s+").length; // 分割线并计算非空格部分的数量
nonSpaceCharCount += line.replaceAll("\\s", "").length(); // 移除所有空格并计算长度
}
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
try (FileWriter writer = new FileWriter(outputFile)) {
writer.write("字符串总数: " + stringCount);
writer.newLine();
writer.write("非空格字符总数: " + nonSpaceCharCount);
} catch (IOException e) {
System.err.println("Error writing to output file: " + e.getMessage());
}
}
```
3. 调用这个方法,传入源文件名和目标文件名:
```java
String sourceFile = "source.txt";
String destFile = "output.txt";
countChars(sourceFile, destFile);
```
阅读全文
相关推荐
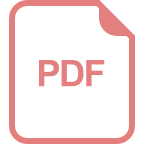
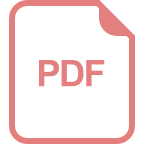
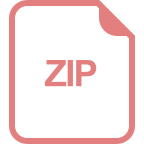

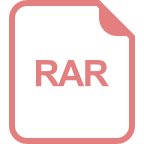
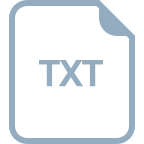
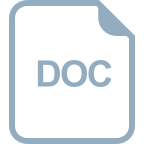
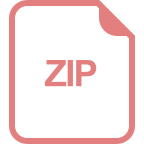
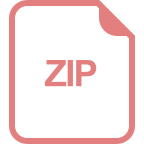
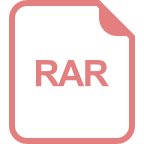
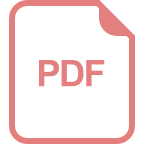
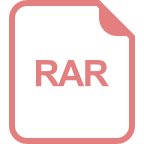
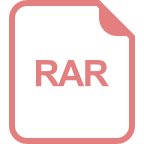
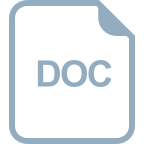