python DS算法实现
时间: 2023-10-31 10:07:27 浏览: 135
DS(数据结构)算法在Python中的实现取决于具体的数据结构和算法。以下是一些常见的数据结构和算法的Python实现示例:
1. 数组(Array):使用Python的列表来表示数组,可以使用索引访问和操作元素。
```python
# 创建一个数组
array = [1, 2, 3, 4, 5]
# 访问数组元素
print(array[0]) # 输出:1
# 修改数组元素
array[0] = 10
# 遍历数组
for element in array:
print(element)
```
2. 链表(Linked List):可以使用自定义的链表类来实现链表数据结构。
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def add_node(self, data):
new_node = Node(data)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def print_list(self):
current = self.head
while current:
print(current.data)
current = current.next
# 创建链表
linked_list = LinkedList()
# 添加节点
linked_list.add_node(1)
linked_list.add_node(2)
linked_list.add_node(3)
# 打印链表
linked_list.print_list()
```
3. 栈(Stack):可以使用Python的列表和一些操作来实现栈数据结构。
```python
# 创建一个空栈
stack = []
# 入栈
stack.append(1)
stack.append(2)
stack.append(3)
# 出栈
top_element = stack.pop()
# 打印栈顶元素
print(top_element)
```
4. 队列(Queue):可以使用Python的collections库中的deque类来实现队列数据结构。
```python
from collections import deque
# 创建一个空队列
queue = deque()
# 入队
queue.append(1)
queue.append(2)
queue.append(3)
# 出队
front_element = queue.popleft()
# 打印队首元素
print(front_element)
```
阅读全文
相关推荐

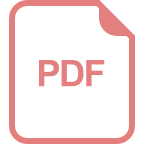
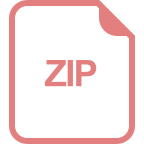
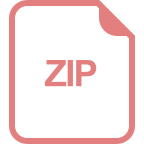
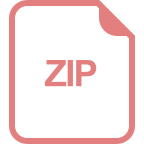
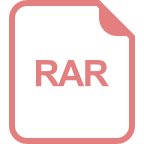
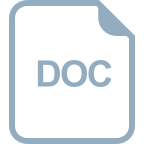

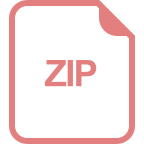
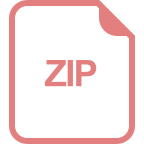
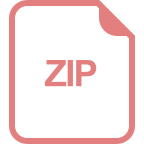
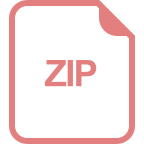
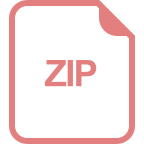
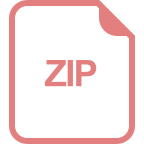