C# 加载bitmap
时间: 2024-08-14 11:00:44 浏览: 58
在C#中,加载Bitmap通常通过System.Drawing命名空间下的Image类来完成。以下是基本步骤:
1. 引入所需命名空间:
```csharp
using System.Drawing;
```
2. 使用`Bitmap.FromFile`方法从文件加载:
```csharp
Bitmap bitmap = new Bitmap("path_to_your_image.jpg");
```
如果你需要从内存读取或流中加载图片,可以使用`FromStream`或`FromMemoryStream`方法。
```csharp
byte[] imageBytes = // 获取图片字节数据
MemoryStream ms = new MemoryStream(imageBytes);
Bitmap bitmap = new Bitmap(ms);
```
或者,如果图片是以URL形式存在:
```csharp
WebClient wc = new WebClient();
string imageUrl = "http://example.com/image.png";
using (var stream = wc.OpenRead(imageUrl))
{
Bitmap bitmap = new Bitmap(stream);
}
```
3. 一旦有了Bitmap实例,你可以对其进行操作,如显示、缩放或保存等。
阅读全文
相关推荐

















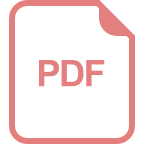
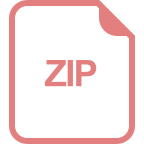
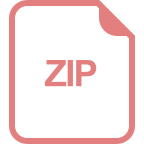