凯撒密码的译码C语言
时间: 2024-09-28 07:01:34 浏览: 43
凯撒密码是一种简单的替换加密技术,它通过将字母表中的每个字符按照固定位移(称为密钥)来进行替换。在C语言中,我们可以创建一个函数来实现凯撒密码的加密和解密。下面是一个基本的凯撒密码编码函数示例:
```c
#include <stdio.h>
#include <string.h>
char caesar_cipher_encrypt(char c, int shift) {
if (c >= 'A' && c <= 'Z') {
return ((c - 'A' + shift) % 26) + 'A';
} else if (c >= 'a' && c <= 'z') {
return ((c - 'a' + shift) % 26) + 'a';
}
// 如果输入不是字母,保持不变
return c;
}
void caesar_cipher(char *message, int shift, char *output, char mode) {
int len = strlen(message);
for (int i = 0; i < len; i++) {
output[i] = mode == 'e' ? caesar_cipher_encrypt(message[i], shift) : caesar_cipher_encrypt(message[i], -shift); // 正向加密或反向解密
}
output[len] = '\0'; // 添加结束符
}
int main() {
char plaintext[50];
char ciphertext[50];
printf("请输入明文:");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strcspn(plaintext, "\n")] = '\0'; // 移除换行符
int key;
printf("请输入密钥:");
scanf("%d", &key);
caesar_cipher(plaintext, key, ciphertext, 'e'); // 加密
printf("加密后的消息是:%s\n", ciphertext);
// 解密
caesar_cipher(ciphertext, key, ciphertext, 'd');
printf("解密后的消息是:%s\n", ciphertext);
return 0;
}
```
在这个程序中,`caesar_cipher_encrypt`函数负责单个字符的加密,`caesar_cipher`函数则遍历整个字符串进行操作。用户会被提示输入明文、密钥以及是否加密还是解密。
阅读全文
相关推荐
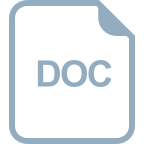
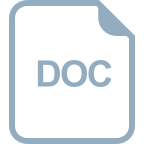
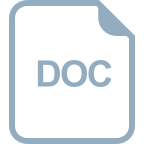
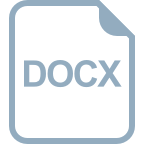
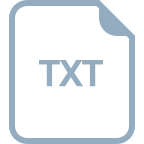
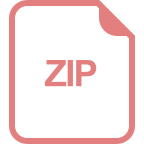
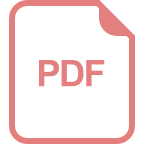
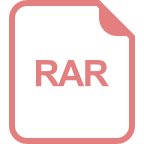
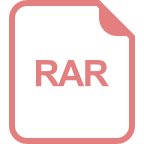
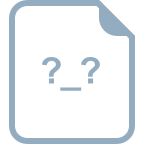





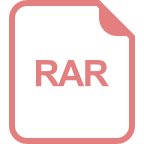
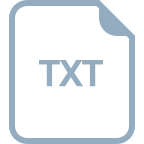