写一个c++调用动态连接库类的调用的例子
时间: 2024-10-24 10:03:21 浏览: 17
在C++中,调用动态链接库(DLL)中的类通常涉及到头文件包含、库的加载以及实例化对象。这里有一个简单的例子,假设我们有一个名为`MyLibrary`的动态库,其中有一个`MyClass`类:
```cpp
#include <iostream>
#include <dlfcn.h>
// 定义 MyClass 的声明
class MyClass;
typedef void (*MyFunction)(int); // 定义函数指针类型
extern "C" {
// DLL 中的导出函数声明
MyClass* createMyObject();
MyFunction myFunction(int);
}
int main() {
// 动态加载库
void* handle = dlopen("libMyLibrary.so", RTLD_LAZY); // Linux
// 或者
// void* handle = LoadLibrary("MyLibrary.dll"); // Windows
if (!handle) {
std::cerr << "Error loading library: " << dlerror() << std::endl; // 或 GetLastError()
return 1;
}
// 获取 MyClass 的定义
MyClass* obj = (MyClass*) dlsym(handle, "createMyObject"); // Linux
// 或者
// MyClass* obj = (MyClass*) GetProcAddress(handle, "createMyObject"); // Windows
if (!obj) {
std::cerr << "Error getting object from library: " << dlerror() << std::endl; // 或 GetLastError()
dlclose(handle); // 关闭已加载的库
return 1;
}
// 调用 DLL 中的函数
MyFunction myFunc = (MyFunction)dlsym(handle, "myFunction");
int result = myFunc(42);
std::cout << "Result from the function: " << result << std::endl;
// 释放对象和关闭库
delete obj;
dlclose(handle);
return 0;
}
```
在这个例子中,你需要将`createMyObject()`和`myFunction(int)`替换为实际的DLL函数名,并确保它们已经用`(dllexport)`声明为可以外部访问。
阅读全文
相关推荐
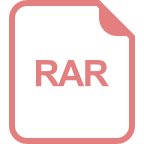
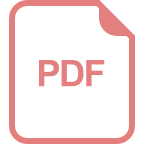
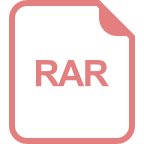
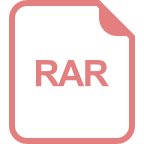
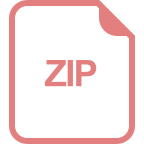
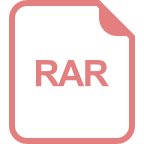
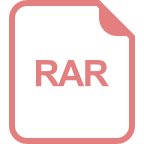
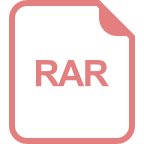
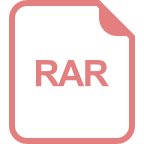
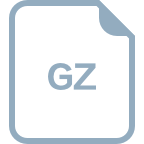
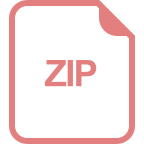
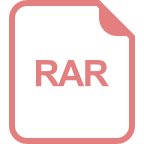
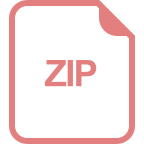
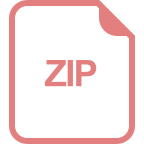
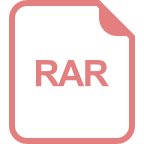
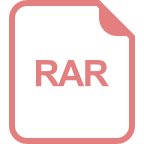