用C语言解决下列问题:输入一个字符串,找出其中指定字符的个数,没有则输出0
时间: 2024-12-20 11:31:03 浏览: 3
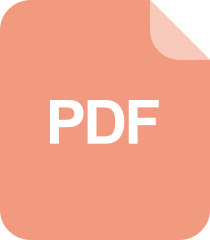
用c语言实现 计算一个字符串中单词的个数

在C语言中,你可以使用标准库函数`strcspn()`和一些基本的循环结构来解决这个问题。`strcspn()`函数返回字符串中从第一个字符到指定位置的第一个不是给定字符集合中的字符的位置。下面是一个简单的示例程序,它会读取用户输入的字符串和指定字符,然后计算指定字符出现的次数:
```c
#include <stdio.h>
#include <string.h>
int count_char(char *str, char target) {
int length = strlen(str);
int count = 0;
for (int i = 0; i < length; i++) {
if (str[i] == target) {
count++;
}
}
return count ? count : 0; // 返回非零值表示找到字符,否则返回0
}
int main() {
char str[100];
printf("请输入一个字符串: ");
fgets(str, sizeof(str), stdin); // 读取字符串,注意去掉行尾的换行符
str[strlen(str)-1] = '\0'; // 因为fgets会多加一个换行符
char target;
printf("请输入你要查找的字符: ");
scanf("%c", &target);
int result = count_char(str, target);
printf("字符 '%c' 在字符串中出现了 %d 次.\n", target, result);
return 0;
}
```
在这个程序中,我们首先获取用户的输入,然后通过`count_char`函数计算目标字符在字符串中出现的次数,并在`main`函数中打印结果。
阅读全文
相关推荐
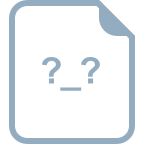
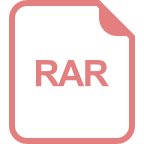















