qt多线程同时执行三个函数并传参
时间: 2023-07-21 19:08:57 浏览: 47
你可以使用Qt的QThread类和信号与槽机制来实现多线程同时执行三个函数并传参的功能。
首先,定义一个继承自QThread的线程类,例如:
```cpp
class MyThread : public QThread
{
Q_OBJECT
public:
explicit MyThread(QObject *parent = nullptr);
void run() override;
signals:
void resultReady(const QString &result);
private:
// 定义需要传递的参数
QString m_param;
};
```
在该类中,我们定义了一个需要传递的参数m_param,并在run函数中执行需要执行的函数,并通过信号resultReady将执行结果传递出来。
接下来,在主线程中创建三个MyThread对象并启动它们,例如:
```cpp
MyThread *thread1 = new MyThread(this);
thread1->m_param = "param1";
connect(thread1, &MyThread::resultReady, this, [](const QString &result){
qDebug() << "Thread1 Result: " << result;
});
thread1->start();
MyThread *thread2 = new MyThread(this);
thread2->m_param = "param2";
connect(thread2, &MyThread::resultReady, this, [](const QString &result){
qDebug() << "Thread2 Result: " << result;
});
thread2->start();
MyThread *thread3 = new MyThread(this);
thread3->m_param = "param3";
connect(thread3, &MyThread::resultReady, this, [](const QString &result){
qDebug() << "Thread3 Result: " << result;
});
thread3->start();
```
在创建三个线程对象并启动它们的过程中,我们将需要传递的参数m_param分别设置为"param1"、"param2"和"param3",并通过connect函数将resultReady信号与对应的槽函数连接起来。
最后,在MyThread类的run函数中执行需要执行的函数,并将执行结果通过resultReady信号传递出来,例如:
```cpp
void MyThread::run()
{
// 执行需要执行的函数
QString result = myFunction(m_param);
// 将执行结果通过信号传递出来
emit resultReady(result);
}
```
其中,myFunction函数为需要执行的函数,通过传递的参数m_param来获取需要的参数。执行结果通过resultReady信号传递出来。
注意:在使用多线程时需要注意线程安全的问题,尤其是在多个线程同时访问同一个资源时,需要保证对该资源的访问是互斥的。可以使用Qt的QMutex类来实现互斥访问。
相关推荐
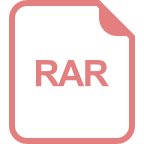
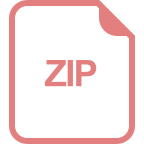
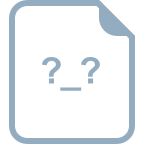














