编写一个长方体类(Cuboid),其数据成员有 (长),width(宽),height(高),两个成员函数分别实现: (1)由键盘分别输入长、宽、高: (2)计算长方体的体积: length (3)为Cuboid添加默认构造函数。 并在主西数中定义该类的一个对象,并测试这些成员函数。
时间: 2024-01-28 21:05:37 浏览: 69
好的,以下是实现长方体类(Cuboid)的代码:
```cpp
#include <iostream>
using namespace std;
class Cuboid {
private:
double length;
double width;
double height;
public:
Cuboid() { // 默认构造函数
length = 0;
width = 0;
height = 0;
}
void input() { // 输入长、宽、高
cout << "请输入长方体的长、宽、高:";
cin >> length >> width >> height;
}
double volume() { // 计算长方体的体积
return length * width * height;
}
};
int main() {
Cuboid c1; // 定义一个长方体对象
c1.input(); // 输入长、宽、高
cout << "长方体的体积为:" << c1.volume() << endl; // 输出长方体的体积
return 0;
}
```
运行程序,你可以输入长、宽、高的值,程序会自动计算长方体的体积并输出。
相关问题
.编写一个长方体类(Cuboid),其数据成员有 length(长),width(宽),height(高),两个成员函数分别实现: (1)由键盘分别输入长、宽、高; (2)计算长方体的体积; (3)仿效教材中例9.1,为Cuboid添加默认构造函数。 并在主函数中定义该类的一个对象,并测试这些成员函数。
好的,根据您的要求,以下是长方体类的实现代码:
```cpp
#include <iostream>
using namespace std;
class Cuboid {
private:
double length;
double width;
double height;
public:
Cuboid() { // 默认构造函数
length = 0;
width = 0;
height = 0;
}
void input() { // 由键盘输入长、宽、高
cout << "请输入长方体的长、宽、高:" << endl;
cin >> length >> width >> height;
}
double volume() { // 计算长方体的体积
return length * width * height;
}
};
int main() {
Cuboid c; // 定义一个长方体对象
c.input(); // 输入长、宽、高
cout << "长方体的体积是:" << c.volume() << endl; // 计算并输出体积
return 0;
}
```
您可以编译运行上述代码,测试它是否符合您的要求。
java编写一个长方体类
好的,可以为您讲解如何编写一个Java长方体类。一个长方体类需要包含长度、宽度和高度等属性,以及计算体积和表面积的方法。以下是一个可能的实现:
```
public class Cuboid {
private double length;
private double width;
private double height;
public Cuboid(double length, double width, double height) {
this.length = length;
this.width = width;
this.height = height;
}
public double getVolume() {
return length * width * height;
}
public double getSurfaceArea() {
return 2 * (length * width + length * height + width * height);
}
}
```
您可以在Java程序中创建一个Cuboid对象,并调用getVolume()和getSurfaceArea()方法来获取长方体的体积和表面积。如下所示:
```
Cuboid cuboid = new Cuboid(4.0, 5.0, 6.0);
double volume = cuboid.getVolume(); // 120.0
double surfaceArea = cuboid.getSurfaceArea(); // 148.0
```
相关推荐
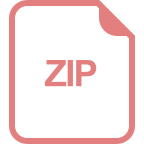
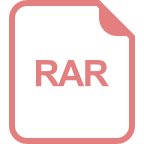












