编写一段代码实现STM32F103R6T6芯片通过两个按键控制一个两位一体共阳极数码管,实现0-99的加减计数(key1控制数码管加计数,key 2控制减计数)
时间: 2024-03-18 12:38:32 浏览: 33
好的,以下是实现该功能的代码:
```c
#include "stm32f10x.h"
// 定义数码管显示的数字和对应的IO口
uint8_t num[10] = {0xc0, 0xf9, 0xa4, 0xb0, 0x99, 0x92, 0x82, 0xf8, 0x80, 0x90};
GPIO_TypeDef* GPIO_PORT[4] = {GPIOA, GPIOB, GPIOB, GPIOB};
const uint16_t GPIO_PIN[4] = {GPIO_Pin_9, GPIO_Pin_5, GPIO_Pin_6, GPIO_Pin_7};
// 计数器变量
uint16_t counter = 0;
// 延时函数
void delay_ms(uint16_t ms) {
uint16_t i,j;
for(i=0;i<ms;i++)
for(j=0;j<1141;j++);
}
// 数码管显示函数
void display(uint16_t num) {
uint8_t data[2] = {num / 10, num % 10};
uint8_t i;
for (i = 0; i < 2; i++) {
GPIO_PORT[i]->ODR &= ~0xFF00;
GPIO_PORT[i]->ODR |= num[data[i]] << 8;
GPIO_PORT[i]->ODR |= GPIO_PIN[i];
delay_ms(5);
GPIO_PORT[i]->ODR &= ~GPIO_PIN[i];
}
}
int main(void) {
// 初始化按键所在的GPIO口
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOC, &GPIO_InitStructure);
// 初始化数码管所在的GPIO口
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 初始显示0
display(counter);
while (1) {
// 检测按键1
if (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_0) == RESET) {
while (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_0) == RESET);
counter++;
if (counter >= 100) {
counter = 0;
}
display(counter);
}
// 检测按键2
if (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_1) == RESET) {
while (GPIO_ReadInputDataBit(GPIOC, GPIO_Pin_1) == RESET);
if (counter == 0) {
counter = 99;
} else {
counter--;
}
display(counter);
}
}
}
```
注:以上代码仅供参考,具体实现方式可能会因芯片型号、外设的连接方式等因素而有所不同,需要根据实际情况进行修改。
相关推荐
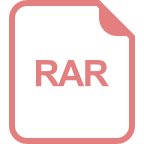
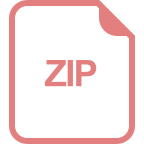














