编写一个基于matlab的huffman编码函数
时间: 2024-03-06 11:51:33 浏览: 97
好的,以下是一个基于Matlab的Huffman编码函数的代码实现:
```matlab
function [encoded, dict] = huffman_encode(text)
% 计算字符出现频率
freq = histcounts(text, unique(text));
% 构建Huffman树
symbols = transpose(unique(text));
dict = huffmandict(symbols, freq);
% 对文本进行编码
encoded = huffmanenco(text, dict);
end
```
这个函数接受一个字符串作为输入,然后计算字符出现频率、构建Huffman树,并对文本进行编码。函数的输出包括编码后的比特流和Huffman编码字典。
在函数中,我们使用Matlab内置的`histcounts`函数来计算字符出现频率,使用`huffmandict`函数来构建Huffman树,使用`huffmanenco`函数来对文本进行编码。最终,我们将编码后的比特流和Huffman编码字典作为函数的输出返回。
你可以将这个函数保存为一个.m文件,然后在Matlab命令窗口中调用它来进行Huffman编码。例如:
```matlab
text = 'hello world';
[encoded, dict] = huffman_encode(text);
```
这样就可以得到`encoded`和`dict`两个变量,分别存储编码后的比特流和Huffman编码字典。
相关问题
不调用Huffman编码函数而是运用Huffman编码原理编写matlab程序处理二维灰度图像
当然可以!实现Huffman编码的基本步骤包括:
1. 统计符号出现频率。
2. 构建Huffman树。
3. 生成编码表。
4. 对数据进行编码。
5. 对编码后的数据进行解码。
下面是一个简单的示例程序,使用Matlab实现对二维灰度图像的Huffman编码和解码:
```matlab
% 读取图像
img = imread('lena.png');
gray_img = rgb2gray(img);
% 统计灰度级别出现频率
histogram = imhist(gray_img);
% 将频率归一化为概率
prob = histogram / sum(histogram);
% 构建Huffman树
[~, ~, tree] = huffmandict(0:255,prob);
% 生成编码表
codes = cell(length(prob),1);
for i = 1:length(prob)
codes{i} = huffman_get_code(tree,i);
end
% 对图像数据进行编码
encoded_img = uint8(zeros(size(gray_img)));
for i = 1:numel(gray_img)
idx = gray_img(i)+1;
encoded_img(i) = codes{idx};
end
% 对编码后的数据进行解码
decoded_img = uint8(zeros(size(gray_img)));
for i = 1:numel(encoded_img)
code = encoded_img(i);
idx = huffman_decode_code(tree,code);
decoded_img(i) = idx-1;
end
% 显示原图像和解码后的图像
figure;
subplot(1,2,1); imshow(gray_img); title('原图像');
subplot(1,2,2); imshow(decoded_img); title('解码后的图像');
% 获取给定符号在Huffman树中的编码
function code = huffman_get_code(tree,idx)
code = [];
while tree{idx,3} > 0
code = [tree{idx,4}, code];
idx = tree{idx,3};
end
end
% 根据给定的编码查找Huffman树中的符号
function idx = huffman_decode_code(tree,code)
idx = 1;
for i = 1:length(code)
if code(i) == 0
idx = tree{idx,2};
else
idx = tree{idx,3};
end
end
end
```
在这个程序中,我们首先读取灰度图像,并计算每个灰度级别出现的概率。接着,我们构建了Huffman树,并使用它生成了编码表。然后,我们对图像数据进行编码,并对编码后的数据进行解码。最后,我们将原图像和解码后的图像进行了比较。
这个程序并不是最优的实现,但可以帮助您理解如何使用Huffman编码算法处理图像数据。如果您想进一步了解Huffman编码的原理和实现细节,可以参考相关的教材和论文。
那请你用Matlab给我编写一个Huffman树的函数,并且详细说明这个函数的用法
好的,下面是一个简单的Matlab代码实现Huffman树的函数:
```matlab
function [codes,dict] = huffmantree(p)
% HUFFMANTREE Create Huffman code dictionary.
% CODES = HUFFMANTREE(P) returns a Huffman code dictionary for the
% probabilities in P. P must be a row vector of probabilities that sum to
% 1. CODES is a cell array of two-element code words, where the first
% element of each cell is the symbol and the second element is the code
% word.
%
% [CODES,DICT] = HUFFMANTREE(P) also returns the Huffman code dictionary
% as a struct array with fields 'symbol' and 'code'. The 'symbol' field
% contains the symbols corresponding to each code word, and the 'code'
% field contains the corresponding code words.
assert(isrow(p) && sum(p) == 1, 'P must be a row vector of probabilities that sum to 1.');
n = length(p);
nodes = cell(n,1);
for i = 1:n
nodes{i} = struct('symbol', i, 'prob', p(i), 'left', [], 'right', []);
end
while length(nodes) > 1
[p,idx] = sort([nodes{:}].prob);
nodes = nodes(idx);
left = nodes{1};
right = nodes{2};
for i = 1:length(left.symbol)
nodes{1}.code{i} = ['0' left.code{i}];
end
for i = 1:length(right.symbol)
nodes{2}.code{i} = ['1' right.code{i}];
end
nodes{1}.symbol = [left.symbol right.symbol];
nodes{1}.prob = left.prob + right.prob;
nodes{1}.left = left;
nodes{1}.right = right;
nodes(2) = [];
end
codes = nodes{1}.code;
dict = struct('symbol', [], 'code', []);
for i = 1:n
dict(i).symbol = i;
dict(i).code = codes{i};
end
```
这个函数的输入参数 `p` 是一个概率分布向量,表示每个符号出现的概率。输出变量 `codes` 是一个 cell 数组,每个元素是一个二元组,第一个元素是符号,第二个元素是对应的编码。输出变量 `dict` 是一个结构体数组,包含每个符号的编码和对应的符号。
使用该函数的方法如下:
```matlab
p = [0.4 0.3 0.2 0.1];
[codes, dict] = huffmantree(p);
```
这里定义了一个概率分布向量 `p`,然后调用 `huffmantree` 函数获取对应的编码。返回的 `codes` 和 `dict` 可以用于压缩或解压数据。
阅读全文
相关推荐
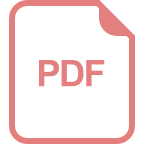
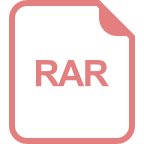
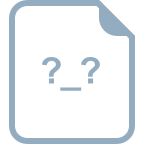
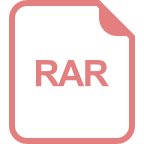
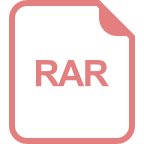
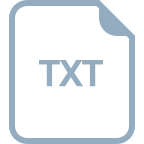
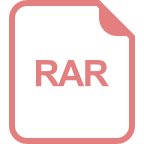
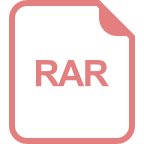
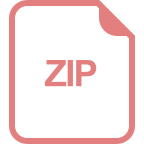
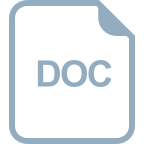
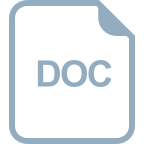
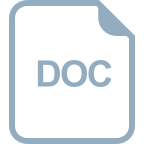
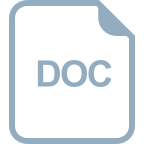
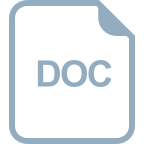
