vue自定义tabs组件
时间: 2023-08-25 13:07:47 浏览: 123
好的,下面是一个简单的自定义tabs组件的实现示例:
```vue
<template>
<div class="tabs">
<div class="tab-header">
<div
v-for="(tab, index) in tabs"
:key="index"
:class="{ active: currentIndex === index }"
@click="changeTab(index)"
>
{{ tab.title }}
</div>
</div>
<div class="tab-content">
<slot />
</div>
</div>
</template>
<script>
export default {
name: "Tabs",
props: {
defaultIndex: {
type: Number,
default: 0,
},
},
data() {
return {
currentIndex: this.defaultIndex,
tabs: [],
};
},
methods: {
changeTab(index) {
this.currentIndex = index;
},
registerTab(tab) {
this.tabs.push(tab);
},
},
mounted() {
this.tabs = this.$children;
},
};
</script>
```
在这个组件中,我们使用了两个插槽,一个是tab-header用于显示标签页标题,另一个是tab-content用于显示标签页内容。组件内部维护了一个tabs数组,用于存储所有的标签页组件。
标签页组件的实现如下:
```vue
<template>
<div v-show="active">
<slot></slot>
</div>
</template>
<script>
export default {
name: "Tab",
props: {
title: {
type: String,
required: true,
},
},
data() {
return {
active: false,
};
},
mounted() {
this.$parent.registerTab(this);
},
updated() {
if (this.$parent.currentIndex === this.$parent.tabs.indexOf(this)) {
this.active = true;
} else {
this.active = false;
}
},
};
</script>
```
在标签页组件中,我们维护了一个active属性,用于控制标签页是否显示。同时,当标签页组件的父组件Tabs更新时,我们会根据当前标签页在tabs数组中的位置和父组件当前激活的标签页的位置来更新active属性。
使用方式如下:
```vue
<template>
<div>
<tabs>
<tab title="Tab 1">
<p>Content of Tab 1</p>
</tab>
<tab title="Tab 2">
<p>Content of Tab 2</p>
</tab>
<tab title="Tab 3">
<p>Content of Tab 3</p>
</tab>
</tabs>
</div>
</template>
<script>
import Tabs from "@/components/Tabs.vue";
import Tab from "@/components/Tab.vue";
export default {
name: "App",
components: {
Tabs,
Tab,
},
};
</script>
```
其中,Tabs组件包含了三个Tab组件,每个Tab组件都有一个标题和内容。可以根据需要自行修改样式和内容。
阅读全文
相关推荐
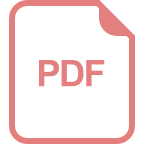
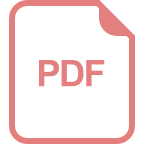
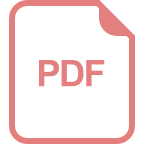
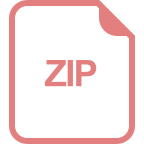
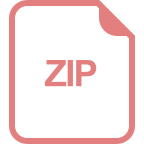
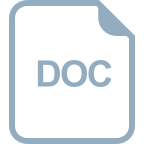
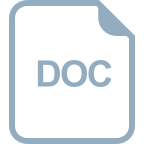


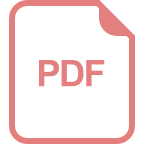
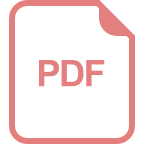
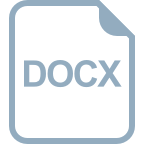
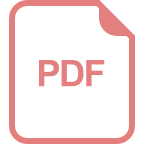


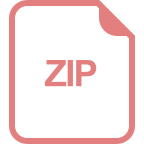