java中编写一个线程类实现文件拷贝
时间: 2023-06-19 15:07:37 浏览: 96
下面是一个简单的Java线程类,实现文件拷贝的功能:
```java
import java.io.*;
public class FileCopyThread extends Thread {
private String sourceFilePath;
private String targetFilePath;
public FileCopyThread(String sourceFilePath, String targetFilePath) {
this.sourceFilePath = sourceFilePath;
this.targetFilePath = targetFilePath;
}
@Override
public void run() {
try {
File sourceFile = new File(sourceFilePath);
File targetFile = new File(targetFilePath);
InputStream in = new FileInputStream(sourceFile);
OutputStream out = new FileOutputStream(targetFile);
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("文件拷贝完成");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用该线程类,可以通过下面的代码来实现文件拷贝:
```java
FileCopyThread copyThread = new FileCopyThread("source.txt", "target.txt");
copyThread.start();
```
在上面的代码中,我们创建了一个FileCopyThread对象,将源文件路径和目标文件路径作为参数传入,然后调用start()方法启动线程。在run()方法中,我们使用InputStream和OutputStream来读取源文件并将其写入目标文件。当文件拷贝完成后,我们输出一条消息来提示用户。
阅读全文
相关推荐
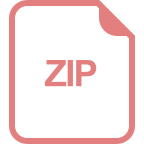
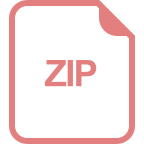
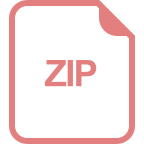
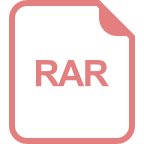
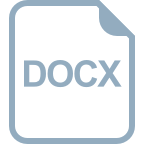
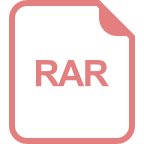
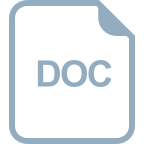
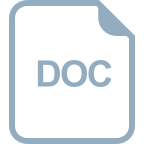
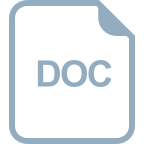
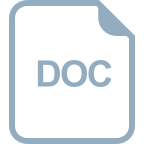
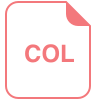
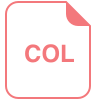
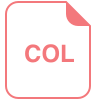
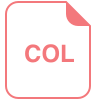
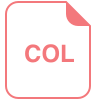


