def zipjie(): import os import pandas as pd # 指定要合并的文件夹路径 folder_path = r"E:\aaaa\aaaa" fields_to_write = ['aaaa', 'aaaa'] # 获取文件夹中所有的 xlsx 文件路径 xlsx_files = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if f.endswith('.xlsx')] # 创建一个空的 DataFrame 用于存储合并后的数据 merged_data = pd.DataFrame() # 循环读取每个 xlsx 文件,将它们合并到 merged_data 中 for xlsx_file in xlsx_files: # 使用 pandas 读取 xlsx 文件 df = pd.read_excel(xlsx_file) # 将读取到的数据追加到 merged_data 中 merged_data = merged_data.append(df, ignore_index=True) # 在 merged_data 中添加新的一列数据 merged_data['new_column'] = 'new_value' # 将合并后的数据写入到新的 xlsx 文件中 merged_data[fields_to_write + ['new_column']].to_excel(r"E:\aaaa\aaaa\merged_file.xlsx", index=False),优化下这条代码,在执行过程中,会出现xml.etree.ElementTree.ParseError: reference to invalid character number: line 1, column 2448367的报错,怎么优化
时间: 2024-03-17 21:39:57 浏览: 132
可以在读取 xlsx 文件时,使用 `openpyxl` 库来修复其中的无效字符引用。以下是一个示例代码:
```python
import os
import pandas as pd
from openpyxl import load_workbook
# 指定要合并的文件夹路径
folder_path = r"E:\aaaa\aaaa"
fields_to_write = ['aaaa', 'aaaa']
# 获取文件夹中所有的 xlsx 文件路径
xlsx_files = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if f.endswith('.xlsx')]
# 创建一个空的 DataFrame 用于存储合并后的数据
merged_data = pd.DataFrame()
# 循环读取每个 xlsx 文件,将它们合并到 merged_data 中
for xlsx_file in xlsx_files:
# 使用 openpyxl 读取 xlsx 文件,并修复其中的无效字符引用
wb = load_workbook(filename=xlsx_file, read_only=True, data_only=True)
ws = wb.active
data = ws.values
headers = next(data)
df = pd.DataFrame(data, columns=headers)
# 将读取到的数据追加到 merged_data 中
merged_data = merged_data.append(df, ignore_index=True)
# 在 merged_data 中添加新的一列数据
merged_data['new_column'] = 'new_value'
# 将合并后的数据写入到新的 xlsx 文件中
merged_data[fields_to_write + ['new_column']].to_excel(r"E:\aaaa\aaaa\merged_file.xlsx", index=False)
```
在这个代码中,我们使用 `load_workbook` 函数从 xlsx 文件中读取数据,并设置 `read_only=True` 和 `data_only=True`,以便只读取数据而不读取公式。然后将其转换为 Pandas DataFrame,并将其追加到 `merged_data` 中。这样可以避免因为无效字符引用而导致的 `xml.etree.ElementTree.ParseError` 错误。
阅读全文
相关推荐
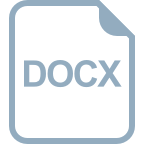
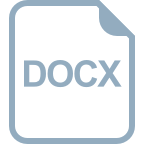
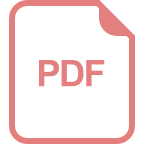


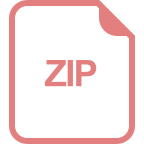
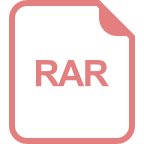
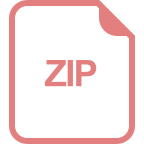
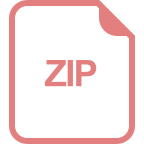
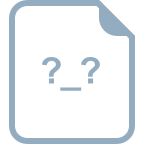
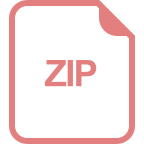
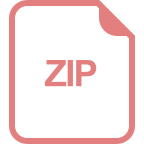
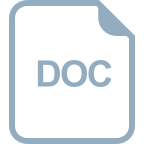