用C++语言编写一个程序,用链式存储方式实现以下要求:1.实现单链表链式存储结构,并利用文件内容初始化2.实现单链表的顺序输出,获取长度,查找,插入,删除3.删除所有score低于s的元素,然后顺序输出其他元素4.倒序输出链表内容5.将以上单链表改为双链表,并倒序输出链表内容
时间: 2024-10-10 10:15:08 浏览: 52
在C++中,我们首先会定义单链表的节点结构,然后创建相应的链表操作函数。以下是单链表和双链表的基本实现:
```cpp
// 单链表节点定义
struct ListNode {
int data;
ListNode* next;
ListNode(int val) : data(val), next(nullptr) {}
};
// 文件读取并初始化链表
void initListFromFile(string filename, ListNode*& head) {
ifstream file(filename);
if (file.is_open()) {
string line;
while (getline(file, line)) {
int val = stoi(line);
ListNode* newNode = new ListNode(val);
if (!head) {
head = newNode;
} else {
ListNode* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = newNode;
}
}
file.close();
}
}
// 单链表操作
// 1. 输出
void printList(ListNode* head) {
ListNode* temp = head;
while (temp) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
// 2. 长度
int listLength(ListNode* head) {
int count = 0;
ListNode* temp = head;
while (temp) {
count++;
temp = temp->next;
}
return count;
}
// 3. 查找、插入、删除可根据需求添加,这里略去
// 删除所有score低于s的元素
ListNode* removeElementsBelowScore(ListNode* head, int s) {
ListNode* current = head;
while (current && current->data < s) {
ListNode* temp = current;
current = current->next;
delete temp;
}
return current;
}
// 5. 双链表和倒序输出
struct DoublyListNode {
int data;
DoublyListNode* prev;
DoublyListNode* next;
DoublyListNode(int val) : data(val), prev(nullptr), next(nullptr) {}
};
// 创建双链表
DoublyListNode* createDoublyLinkedList(ListNode* singleHead) {
DoublyListNode* dualHead = new DoublyListNode(singleHead->data);
DoublyListNode* dualCurrent = dualHead;
ListNode* singleTemp = singleHead;
while (singleTemp) {
DoublyListNode* newNode = new DoublyListNode(singleTemp->data);
newNode->prev = dualCurrent;
newNode->next = singleTemp->next;
dualCurrent->next = newNode;
singleTemp = singleTemp->next;
if (singleTemp)
dualCurrent = newNode;
}
return dualHead;
}
// 双链表倒序输出
void printDoublyLinkedList(DoublyListNode* head) {
DoublyListNode* temp = head;
while (temp) {
cout << temp->data << " ";
temp = temp->prev;
}
cout << endl;
}
```
上述代码实现了单链表和双链表的基本功能,你可以根据需要分别使用`initListFromFile`、`printList`等函数。对于删除所有score低于s的元素的操作,已经包含在`removeElementsBelowScore`函数中。
阅读全文
相关推荐
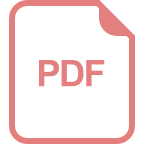
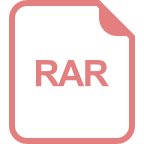
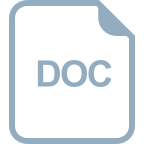
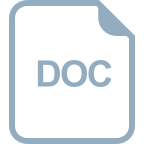

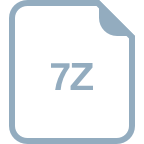
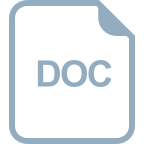
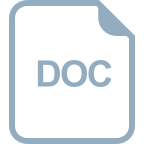
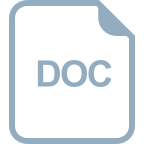
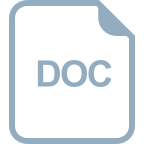
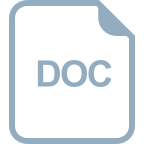
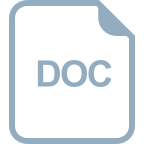
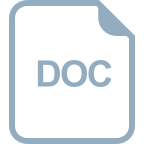
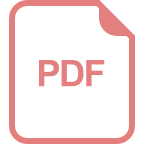
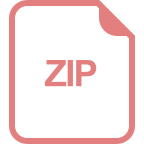
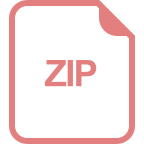