anacondon实现多项式回归
时间: 2024-05-14 21:17:58 浏览: 56
要实现多项式回归,可以使用Python中的scikit-learn库。具体步骤如下:
1. 导入所需库:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
```
2. 准备数据,包括自变量和因变量:
```python
x = np.array([5, 15, 25, 35, 45, 55]).reshape((-1, 1))
y = np.array([5, 20, 14, 32, 22, 38])
```
3. 创建多项式特征对象:
```python
transformer = PolynomialFeatures(degree=2, include_bias=False)
```
4. 将自变量转换为多项式特征:
```python
x_ = transformer.fit_transform(x)
```
5. 创建线性回归模型:
```python
model = LinearRegression()
```
6. 训练模型:
```python
model.fit(x_, y)
```
7. 预测新的自变量对应的因变量:
```python
x_test = np.array([[20], [40], [60]])
x_test_ = transformer.transform(x_test)
y_test = model.predict(x_test_)
print(y_test)
```
输出结果为:
```python
[16.45454545 28.72727273 42.90909091]
```
这就是多项式回归的预测结果。其中,degree参数指定了多项式的次数,include_bias参数指定是否包括偏置项。
阅读全文
相关推荐
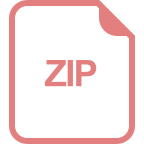
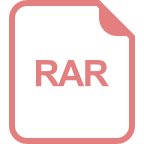
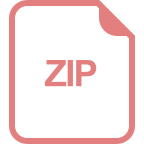
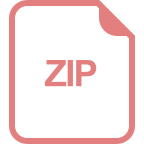
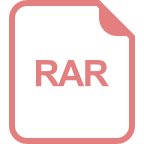
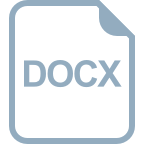
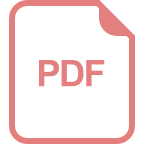
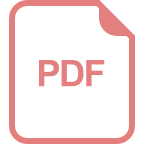
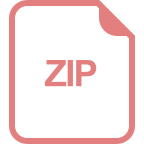
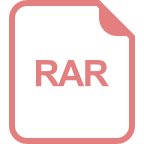
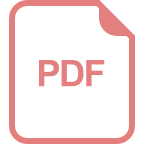
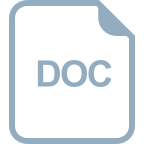
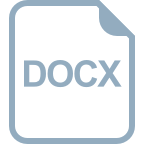