有一个数组,元素为线段,将数组内处于相交状态的线段放入迭代器,用扫描线算法实现
时间: 2024-03-20 15:44:18 浏览: 55
好的,我可以帮你解答这个问题。
扫描线算法是一种常用的解决线段相交问题的方法,具体思路如下:
1. 将所有线段的端点按照 x 坐标从小到大排序。
2. 从左往右扫描线段,将每个线段的左右端点插入到一个有序集合中(例如红黑树)。
3. 每次插入一个端点后,判断该端点是否与相邻的线段有交点,如果有则将它们加入迭代器中。
4. 在有序集合中删除该线段的左端点,如果该线段的右端点还在集合中,则继续判断它与相邻线段是否有交点。
下面是 C++ 代码实现,其中 Line 是表示线段的结构体。
```c++
#include <algorithm>
#include <set>
#include <vector>
using namespace std;
struct Point {
int x, y;
};
struct Line {
Point a, b;
};
bool cmp(const Line& l1, const Line& l2) {
return l1.a.x < l2.a.x;
}
bool intersect(const Line& l1, const Line& l2) {
int c1 = (l1.b.x - l1.a.x) * (l2.a.y - l1.a.y) - (l1.b.y - l1.a.y) * (l2.a.x - l1.a.x);
int c2 = (l1.b.x - l1.a.x) * (l2.b.y - l1.a.y) - (l1.b.y - l1.a.y) * (l2.b.x - l1.a.x);
int c3 = (l2.b.x - l2.a.x) * (l1.a.y - l2.a.y) - (l2.b.y - l2.a.y) * (l1.a.x - l2.a.x);
int c4 = (l2.b.x - l2.a.x) * (l1.b.y - l2.a.y) - (l2.b.y - l2.a.y) * (l1.b.x - l2.a.x);
return (c1 * c2 < 0) && (c3 * c4 < 0);
}
vector<Line> findIntersect(vector<Line>& lines) {
vector<Line> res;
set<pair<int, int>> endpoints; // 存储端点的集合,按照 x 坐标从小到大排序
sort(lines.begin(), lines.end(), cmp);
for (auto& line : lines) {
auto left = endpoints.lower_bound(make_pair(line.a.x, line.a.y));
auto right = endpoints.upper_bound(make_pair(line.b.x, line.b.y));
for (auto it = left; it != right; ++it) {
if (intersect(line, {it->first, it->second, it->first+1, it->second+1})) {
res.push_back({{it->first, it->second}, {line.a.x, line.a.y}});
}
}
endpoints.erase(left, right);
endpoints.insert(make_pair(line.a.x, line.a.y));
endpoints.insert(make_pair(line.b.x, line.b.y));
}
return res;
}
```
其中,cmp 函数是用来排序的比较函数,intersect 函数是判断两条线段是否相交的函数,findIntersect 函数是主要的扫描线算法实现函数。
希望这个解答能够帮到你,如果还有什么问题可以继续问我。
阅读全文
相关推荐
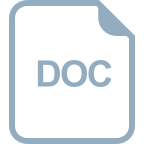
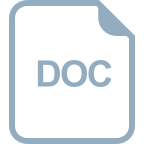
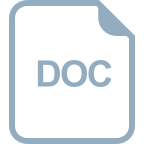


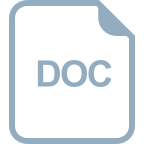
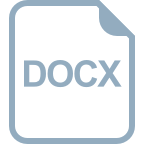
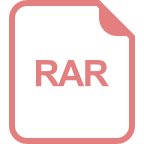
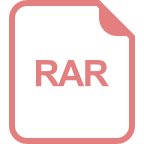
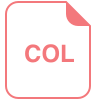
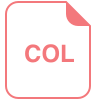
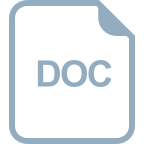
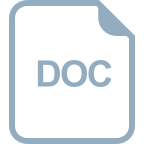
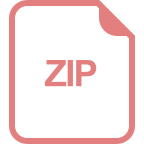
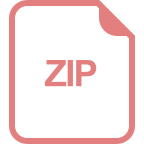
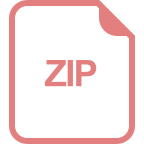
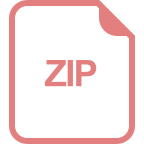
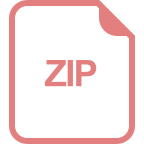