用MFC开发一个登陆界面
时间: 2024-06-12 16:08:17 浏览: 151
这里提供一个简单的MFC登陆界面的代码实现,仅供参考:
1. 创建一个MFC工程,选择对话框模板。
2. 在资源视图中,双击IDD_LOGIN对话框,打开对话框编辑器。
3. 拖拽三个静态文本控件和两个编辑框控件到对话框上,并设置相应的属性。例如,设置静态文本控件的标题、编辑框控件的ID等等。
4. 拖拽一个按钮控件到对话框上,并设置相应的属性。例如,设置按钮控件的标题、ID等等。
5. 双击按钮控件,在按钮的响应函数OnBnClicked()中实现登陆逻辑。例如,判断输入的用户名和密码是否正确,如果正确则跳转到主界面;否则弹出错误提示框。
以下是示例代码:
// LoginDlg.cpp : implementation file
//
#include "stdafx.h"
#include "Login.h"
#include "LoginDlg.h"
#include "afxdialogex.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialogEx
{
public:
CAboutDlg();
// Dialog Data
enum { IDD = IDD_ABOUTBOX };
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
// Implementation
protected:
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialogEx(CAboutDlg::IDD)
{
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialogEx::DoDataExchange(pDX);
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialogEx)
END_MESSAGE_MAP()
// CLoginDlg dialog
CLoginDlg::CLoginDlg(CWnd* pParent /*=NULL*/)
: CDialogEx(CLoginDlg::IDD, pParent)
, m_strUsername(_T(""))
, m_strPassword(_T(""))
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CLoginDlg::DoDataExchange(CDataExchange* pDX)
{
CDialogEx::DoDataExchange(pDX);
DDX_Text(pDX, IDC_EDIT_USERNAME, m_strUsername);
DDX_Text(pDX, IDC_EDIT_PASSWORD, m_strPassword);
}
BEGIN_MESSAGE_MAP(CLoginDlg, CDialogEx)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(IDC_BUTTON_LOGIN, &CLoginDlg::OnBnClickedButtonLogin)
END_MESSAGE_MAP()
// CLoginDlg message handlers
BOOL CLoginDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
return TRUE; // return TRUE unless you set the focus to a control
}
void CLoginDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == SC_CLOSE)
{
// TODO: Add your message handler code here and/or call default
CDialogEx::OnSysCommand(nID, lParam);
if (MessageBox(_T("Are you sure you want to exit?"), _T("Exit"), MB_YESNO | MB_ICONQUESTION) == IDYES)
{
PostQuitMessage(0);
}
}
else
{
CDialogEx::OnSysCommand(nID, lParam);
}
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CLoginDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialogEx::OnPaint();
}
}
// The system calls this function to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CLoginDlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CLoginDlg::OnBnClickedButtonLogin()
{
// TODO: Add your control notification handler code here
UpdateData(TRUE);
if (m_strUsername.IsEmpty() || m_strPassword.IsEmpty())
{
MessageBox(_T("Please input username and password."), _T("Error"), MB_OK | MB_ICONERROR);
}
else if (m_strUsername == _T("admin") && m_strPassword == _T("123456"))
{
MessageBox(_T("Login succeeded."), _T("Success"), MB_OK | MB_ICONINFORMATION);
EndDialog(IDOK);
}
else
{
MessageBox(_T("Username or password is incorrect."), _T("Error"), MB_OK | MB_ICONERROR);
}
}
阅读全文
相关推荐
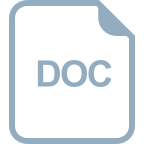
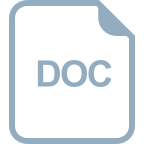
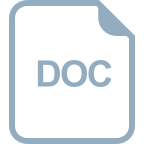
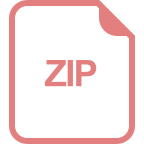
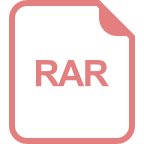
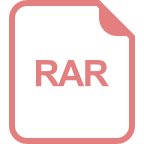
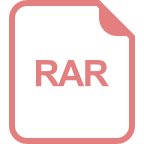
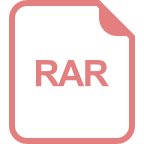
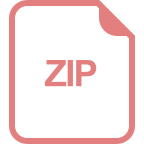
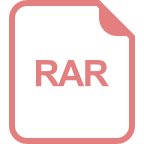
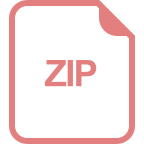

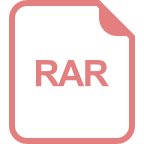
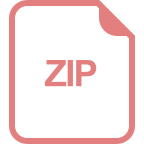
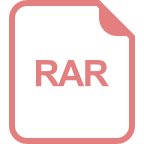
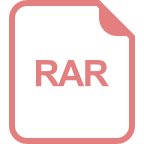
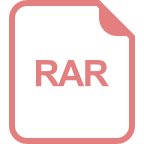
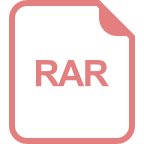
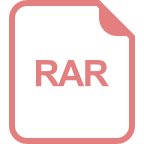