Android将界面输入int型数据存入MySQL
时间: 2024-05-04 09:19:02 浏览: 15
要将Android界面输入的int类型数据存储到MySQL数据库中,需要进行以下步骤:
1. 在Android应用程序中,获取用户输入的int类型数据,并将其存储在一个变量中。
2. 创建一个Java类,用于连接到MySQL数据库并执行SQL语句。 在该类中,您可以使用JDBC API来连接到MySQL数据库。 您需要设置MySQL JDBC驱动程序,并指定MySQL数据库的URL,用户名和密码。
3. 在Java类中,编写SQL语句将int类型数据插入MySQL数据库中的表中。 SQL语句应该包括表名和列名,以及要插入的值。
4. 在Android应用程序中,调用Java类中的方法来连接到MySQL数据库并执行SQL语句。
下面是一个简单的示例代码,用于将int类型数据插入到MySQL数据库中:
```
//获取用户输入的int类型数据
int inputValue = 123;
//连接到MySQL数据库并执行SQL语句
Connection conn = null;
PreparedStatement stmt = null;
try {
//设置MySQL JDBC驱动程序
Class.forName("com.mysql.jdbc.Driver");
//连接到MySQL数据库
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
conn = DriverManager.getConnection(url, user, password);
//编写SQL语句将int类型数据插入MySQL数据库中的表中
String sql = "INSERT INTO mytable (column_name) VALUES (?)";
stmt = conn.prepareStatement(sql);
stmt.setInt(1, inputValue);
stmt.executeUpdate();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
```
在上面的代码中,mydatabase是MySQL数据库的名称,root是MySQL数据库的用户名,mypassword是MySQL数据库的密码,mytable是要插入数据的表名,column_name是要插入数据的列名,inputValue是要插入的int类型数据的变量名。
相关推荐
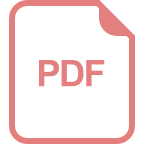
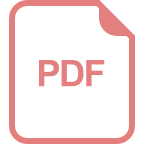
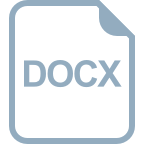














