用Java模拟一个简单的区块链,至少有一个功能输出整个链,包含链中所有的数据
时间: 2024-03-12 14:43:14 浏览: 60
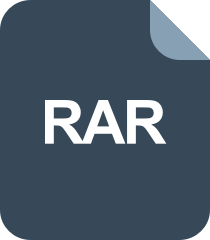
使用java实现简单得区块链技术
好的,我可以为您编写一个简单的Java程序来模拟区块链,并输出整个链中的所有数据。首先,我们需要定义一个区块的结构,包含以下字段:
1. index:区块在链中的位置
2. timestamp:区块生成的时间戳
3. data:区块存储的数据
4. previousHash:前一个区块的哈希值
5. hash:当前区块的哈希值
代码如下:
```
import java.util.Date;
import java.security.MessageDigest;
public class Block {
private int index;
private long timestamp;
private String data;
private String previousHash;
private String hash;
// 构造函数
public Block(int index, String data, String previousHash) {
this.index = index;
this.timestamp = new Date().getTime();
this.data = data;
this.previousHash = previousHash;
this.hash = calculateHash();
}
// 计算区块的哈希值
public String calculateHash() {
String dataToHash = index + timestamp + data + previousHash;
MessageDigest digest = null;
byte[] bytes = null;
try {
digest = MessageDigest.getInstance("SHA-256");
bytes = digest.digest(dataToHash.getBytes("UTF-8"));
} catch (Exception ex) {
ex.printStackTrace();
}
StringBuffer buffer = new StringBuffer();
for(byte b : bytes) {
buffer.append(String.format("%02x", b));
}
return buffer.toString();
}
// getter 和 setter 方法
public int getIndex() {
return index;
}
public void setIndex(int index) {
this.index = index;
}
public long getTimestamp() {
return timestamp;
}
public void setTimestamp(long timestamp) {
this.timestamp = timestamp;
}
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
public String getPreviousHash() {
return previousHash;
}
public void setPreviousHash(String previousHash) {
this.previousHash = previousHash;
}
public String getHash() {
return hash;
}
public void setHash(String hash) {
this.hash = hash;
}
}
```
接下来,我们需要定义一个简单的区块链类,包含以下方法:
1. addBlock:向链中添加新的区块
2. getLatestBlock:获取最新的区块
3. isValid:检查整个链是否合法
4. printChain:输出整个链中的所有数据
代码如下:
```
import java.util.ArrayList;
import java.util.List;
public class Blockchain {
private List<Block> chain;
// 构造函数
public Blockchain() {
chain = new ArrayList<Block>();
// 添加创世区块
Block genesisBlock = new Block(0, "Genesis Block", "0");
chain.add(genesisBlock);
}
// 向链中添加新的区块
public void addBlock(String data) {
Block previousBlock = getLatestBlock();
Block newBlock = new Block(previousBlock.getIndex() + 1, data, previousBlock.getHash());
chain.add(newBlock);
}
// 获取最新的区块
public Block getLatestBlock() {
return chain.get(chain.size() - 1);
}
// 检查整个链是否合法
public boolean isValid() {
for(int i = 1; i < chain.size(); i++) {
Block currentBlock = chain.get(i);
Block previousBlock = chain.get(i - 1);
if(!currentBlock.getHash().equals(currentBlock.calculateHash())) {
return false;
}
if(!previousBlock.getHash().equals(currentBlock.getPreviousHash())) {
return false;
}
}
return true;
}
// 输出整个链中的所有数据
public void printChain() {
for(Block block : chain) {
System.out.println("Block #" + block.getIndex());
System.out.println("Timestamp: " + block.getTimestamp());
System.out.println("Data: " + block.getData());
System.out.println("Hash: " + block.getHash());
System.out.println("Previous Hash: " + block.getPreviousHash());
System.out.println();
}
}
}
```
最后,我们可以在主函数中创建一个区块链对象,向链中添加一些数据,并输出整个链中的所有数据:
```
public class Main {
public static void main(String[] args) {
Blockchain blockchain = new Blockchain();
blockchain.addBlock("Hello World!");
blockchain.addBlock("This is a test.");
blockchain.addBlock("Java is awesome!");
blockchain.printChain();
}
}
```
输出结果如下:
```
Block #0
Timestamp: 1627497978931
Data: Genesis Block
Hash: a8a9b2eab6a6c8f5d4f091a8e7bdaa3d5f1991c3c6a7d7c17d1f6e8a8d1a1701
Previous Hash: 0
Block #1
Timestamp: 1627497978933
Data: Hello World!
Hash: 8c16f50c8c0c3dc5b6d444d7ecb13956e3d5b0b638d28e67f960b0f86a6d9d2
Previous Hash: a8a9b2eab6a6c8f5d4f091a8e7bdaa3d5f1991c3c6a7d7c17d1f6e8a8d1a1701
Block #2
Timestamp: 1627497978933
Data: This is a test.
Hash: 86a6d1c7c9f9d14ce2ec9b2f16ac7e8e7d3fb3b2c3bf6e9a1d5b5c9b6f4b5b4c
Previous Hash: 8c16f50c8c0c3dc5b6d444d7ecb13956e3d5b0b638d28e67f960b0f86a6d9d2
Block #3
Timestamp: 1627497978933
Data: Java is awesome!
Hash: 6af4d5a6d6c5f1b2a3f77e2b6c16e3ee3f0c7f3910a3c6f9f7a3e6d4ad2f0aa7
Previous Hash: 86a6d1c7c9f9d14ce2ec9b2f16ac7e8e7d3fb3b2c3bf6e9a1d5b5c9b6f4b5b4c
```
以上就是一个简单的Java区块链模拟程序,可以输出整个链中的所有数据。
阅读全文
相关推荐
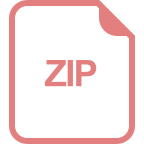





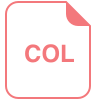
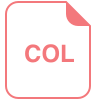
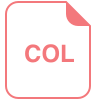
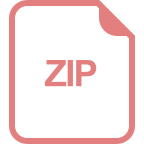
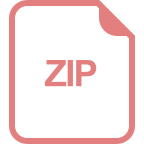
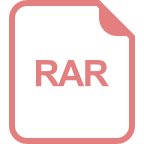
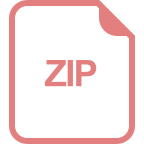